Are you looking for an answer to the topic “typescript cast object to type“? We answer all your questions at the website Chambazone.com in category: Blog sharing the story of making money online. You will find the answer right below.
Keep Reading

How do I cast objects in TypeScript?
- let input = document.querySelector(‘input[“type=”text”]’); …
- console.log(input.value); …
- let input = document.querySelector(‘input[type=”text”]’) as HTMLInputElement; …
- console.log(input.value); …
- let enteredText = (input as HTMLInputElement).value;
Is type casting bad in TypeScript?
Typescript is about typings, but you always have a way to break the typing for good or bad reasons. So here are some key advices. Always type (not cast) all your code but forget the any as much as possible. If you have to cast, it’s a polymorphism-like reason, or it’s probably a code smell.
How to cast type from an observable object to an Interface in angular? TypeScript typecasting
Images related to the topicHow to cast type from an observable object to an Interface in angular? TypeScript typecasting

How do you cast an object in JavaScript?
- const person = { firstName: ‘John’, lastName: ‘Doe’ }; …
- const propertyNames = Object.keys(person); console.log(propertyNames); …
- const propertyValues = Object.values(person); console.log(propertyValues); …
- const entries = Object.entries(person); console.log(entries);
What is casting a data type?
Type casting refers to changing an variable of one data type into another. The compiler will automatically change one type of data into another if it makes sense. For instance, if you assign an integer value to a floating-point variable, the compiler will convert the int to a float.
What is type casting in JavaScript?
In programming language, casting is a way of telling the compiler to change an expression or value from one type to another. At times, you may want to convert your JavaScript expressions or values from one type to another.
How do I cast an Array in TypeScript?
There are 4 possible conversion methods in TypeScript for arrays: let x = []; //any[] let y1 = x as number[]; let z1 = x as Array<number>; let y2 = <number[]>x; let z2 = <Array<number>>x; The as operator’s mostly designed for *.
Is casting code smell?
Explicit casts. I wish there was a compiler option that could make it flag these as warnings (or even better, errors). These hateful things sit there like timebombs in your code, just waiting for an opportunity to explode in a shower of ClassCastExceptions.
See some more details on the topic typescript cast object to type here:
Type Casting – TypeScript Tutorial
JavaScript doesn’t have a concept of type casting because variables have dynamic types. However, every variable in TypeScript has a type.
How Cast Object Works in TypeScript? – eduCBA
The typescript cast object is one of the features for converting the one type of variable values. Data types may be integer, string, float, double values or …
cast an object to other type. How? And instanceof or typeof?
The instanceof operator requires the left operand to be of type Any, an object type, or a type parameter type, and the right operand to be of …
Typescript cast is a type breaker – DEV Community
Typescript offers the “cast” feature to convert from a type to another. But using it wrong can break the type-check of your code.
How do you check if an object has a property in TypeScript?
The first way is to invoke object. hasOwnProperty(propName) . The method returns true if the propName exists inside object , and false otherwise.
How do I convert a string to a number in TypeScript?
In typescript, there are numerous ways to convert a string to a number. We can use the ‘+’ unary operator , Number(), parseInt() or parseFloat() function to convert string to number.
How do you turn an object into a string?
We can convert Object to String in java using toString() method of Object class or String. valueOf(object) method. You can convert any object to String in java whether it is user-defined class, StringBuilder, StringBuffer or anything else.
How do you Stringify an object?
Stringify a JavaScript Object
Use the JavaScript function JSON. stringify() to convert it into a string. const myJSON = JSON. stringify(obj);
How do you cast a class in JavaScript?
- class Person {
- constructor(obj) {
- obj && Object. assign(this, obj);
- }
-
- getFullName() {
- return `${this. lastName} ${this. firstName}`;
- }
TypeScript Basics 30 – Convert response to model object
Images related to the topicTypeScript Basics 30 – Convert response to model object

Is type casting bad?
Overall, being “type-cast” is not necessarily a bad thing, in fact it helps Hollywood produce quality movies. Actors and actresses hone a craft and make a certain type of character and role theirs and it improves the movies they make.
How do you do type casting?
Typecasting is making a variable of one type, such as an int, act like another type, a char, for one single operation. To typecast something, simply put the type of variable you want the actual variable to act as inside parentheses in front of the actual variable. (char)a will make ‘a’ function as a char.
Is type casting and type conversion same?
1. In type casting, a data type is converted into another data type by a programmer using casting operator. Whereas in type conversion, a data type is converted into another data type by a compiler.
Does JavaScript support type casting?
Converting a data type into another is known as type casting. Sometimes there is a need to convert the data type of one value to another. Under some circumstances JavaScript will perform automatic type conversion.
How do you convert something to a string in JavaScript?
The toString() method in Javascript is used with a number and converts the number to a string. It is used to return a string representing the specified Number object. The toString() method is used with a number num as shown in above syntax using the ‘.
What is == and === in JavaScript?
= is used for assigning values to a variable in JavaScript. == is used for comparison between two variables irrespective of the datatype of variable. === is used for comparision between two variables but this will check strict type, which means it will check datatype and compare two values.
How do I Stringify a JSON object in TypeScript?
- Use the JSON.stringify() Method to Convert an Object Into a JSON String in TypeScript.
- Use JSON.stringify() and JSON.parse() to Convert an Object Into a JSON String in TypeScript.
How do you make an array into a string?
- Arrays. toString() method: Arrays. toString() method is used to return a string representation of the contents of the specified array. …
- StringBuilder append(char[]): The java. lang. StringBuilder.
How do you access an array of objects?
A nested data structure is an array or object which refers to other arrays or objects, i.e. its values are arrays or objects. Such structures can be accessed by consecutively applying dot or bracket notation. Here is an example: const data = { code: 42, items: [{ id: 1, name: ‘foo’ }, { id: 2, name: ‘bar’ }] };
Is casting inefficient?
No, casts are fast – as little as a single instruction. The cast itself is not your problem. micros() returns an unsigned long. Any math done on the return value with less than an unsigned long is highly likely to give an incorrect result due to truncation and/or wrap-around.
TypeScript Tutorial #11 – The DOM Type Casting
Images related to the topicTypeScript Tutorial #11 – The DOM Type Casting
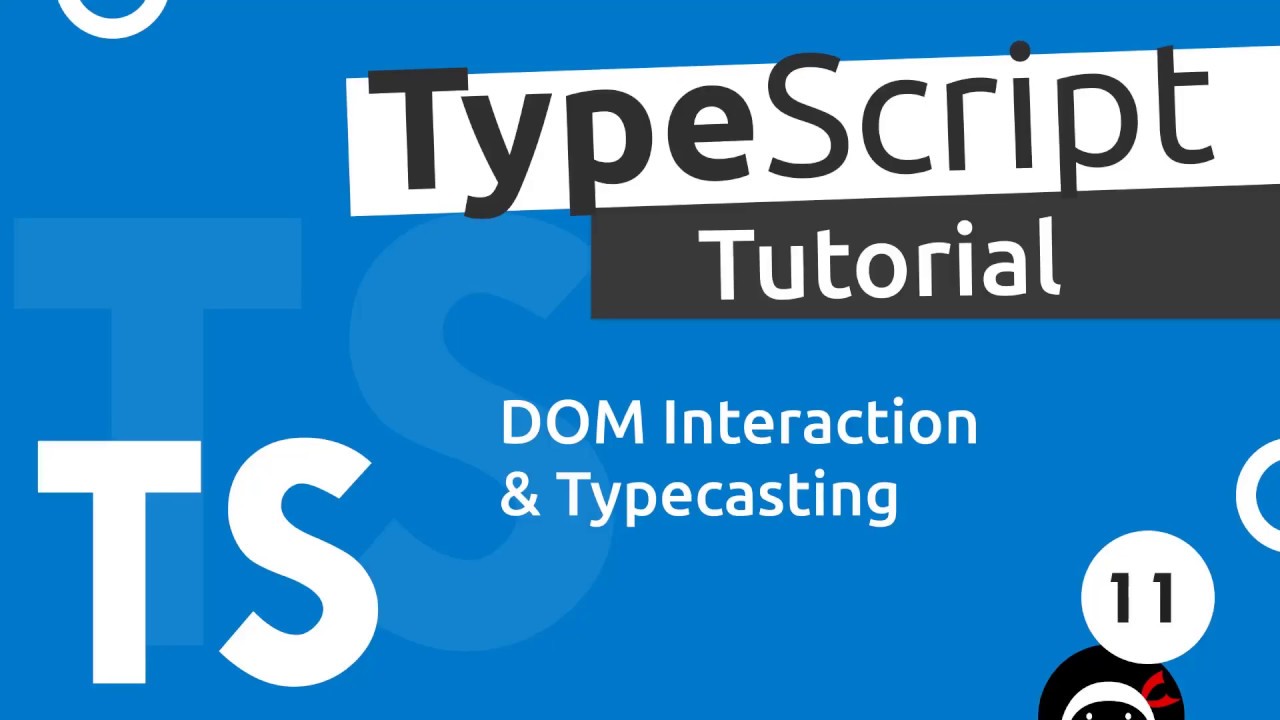
Which does not require type casting?
char c; int i = 65; c = i; There is no need for a cast in this assignment because the integer type int of variable i is implicitly converted to the integer type of the destination.
Why do cast sometimes lead to erroneous code?
This error occurs because the cast is not preserved all the way through the C++ code generation (which occurs at application compile time).
Related searches to typescript cast object to type
- typescript cast unknown to object
- typescript cast object property
- typescript convert object to another object
- typescript cast object to interface
- typescript cast object literal to type
- typescript or type cast
- typescript check if object can be cast to type
- typescript cast object to another type
- typescript cast object to map
- typescript types in object
- typescript cast json object to type
- typescript cast object to different type
- typescript cast object array to type array
- typescript cast object to class type
- typescript cast interface to class
- typescript cast object to generic type
- typescript cast any to type
- how to give object type in typescript
- typescript cast to type
Information related to the topic typescript cast object to type
Here are the search results of the thread typescript cast object to type from Bing. You can read more if you want.
You have just come across an article on the topic typescript cast object to type. If you found this article useful, please share it. Thank you very much.