Are you looking for an answer to the topic “typescript clone array“? We answer all your questions at the website Chambazone.com in category: Blog sharing the story of making money online. You will find the answer right below.
Keep Reading
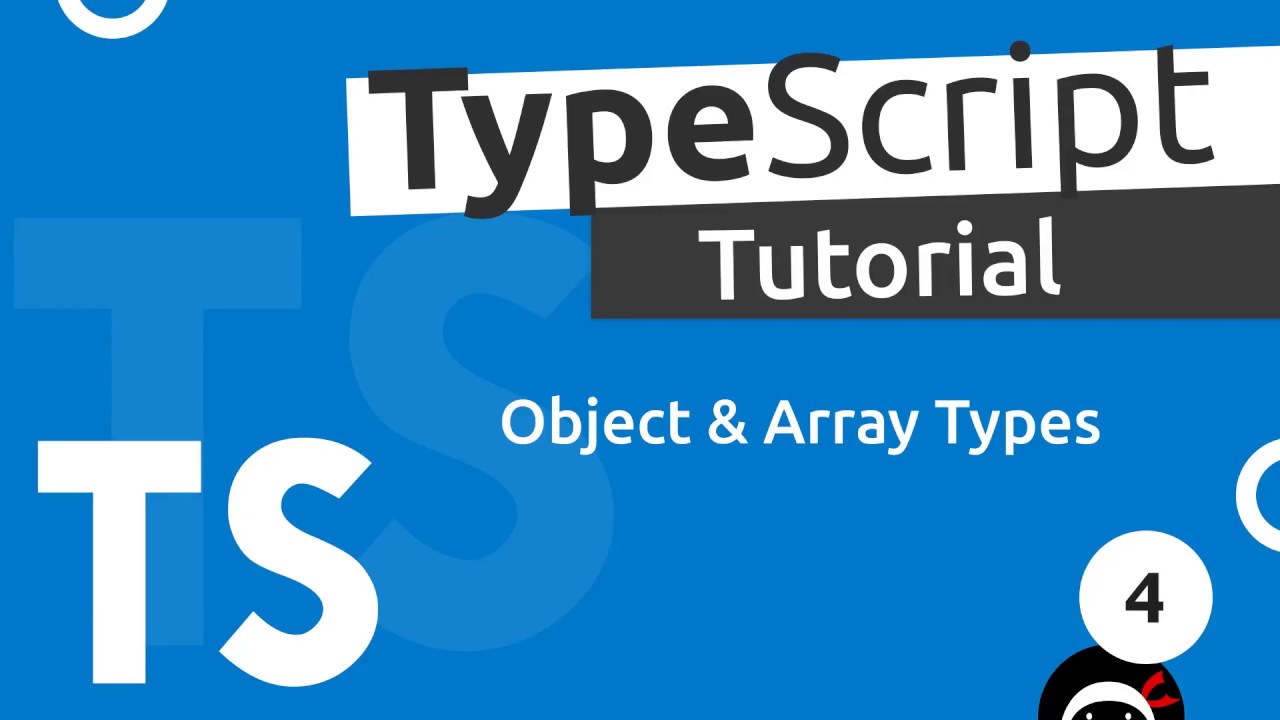
How do I create a copy of an array in TypeScript?
- let arr =[“a”,”b”,”c”];
- const copy = [… arr];
- const copy = Array. from(arr);
How do I clone an array?
If you want to copy the first few elements of an array or a full copy of an array, you can use Arrays. copyOf() method. Arrays. copyOfRange() is used to copy a specified range of an array.
TypeScript Tutorial #4 – Objects Arrays
Images related to the topicTypeScript Tutorial #4 – Objects Arrays
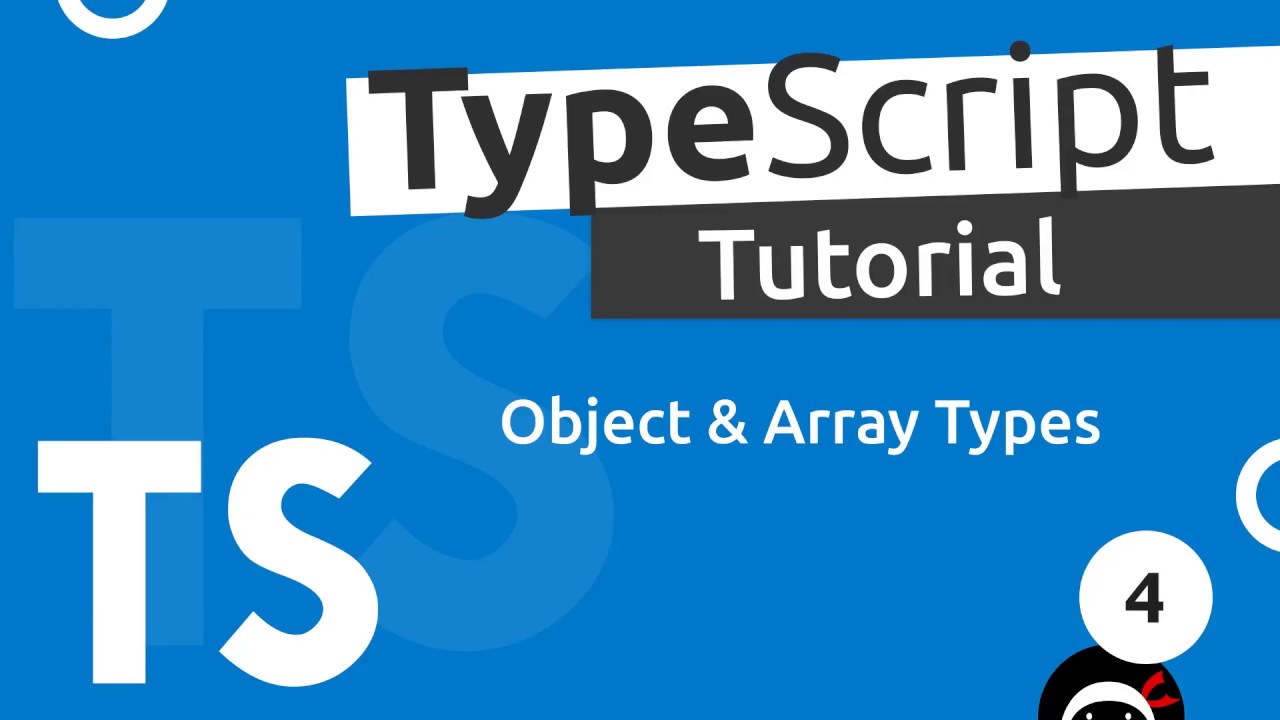
How do you clone an array in Javascript?
- Using Modern ES6 Spread Operator. This is the modern method to clone an array in Javascript. …
- Using Slice. This is yet another popular way to copy an array in Javascript. …
- Using Concat. This method is another popular way to copy an array in Javascript.
How do you copy values from an array in TypeScript?
- /* Copying arrays or parts of arrays in JavaScript */
-
- var fruit = [“apple”, “banana”, “fig”]; // Define initial array.
- console. log(fruit); // [“apple”, “banana”, “fig”]
-
- // Copy an entire array using .slice()
- var fruit2 = fruit. slice();
- console.
How do you copy an object in TypeScript?
Use the Object. assign() Method to Clone an Object in TypeScript. The Object. assign() works similarly as in the case of the spread operator and can be used to clone simple objects, but it fails in the case of nested objects.
How do you push data from one array to another array in TypeScript?
Use the concat function, like so: var arrayA = [1, 2]; var arrayB = [3, 4]; var newArray = arrayA. concat(arrayB); The value of newArray will be [1, 2, 3, 4] ( arrayA and arrayB remain unchanged; concat creates and returns a new array for the result).
How do I make a deep copy of an ArrayList?
To create a true deep copy of ArrayList, we should create a new ArrayList and copy all the cloned elements to new ArrayList one by one and we should also clone Student object properly. To create deep copy of Student class, we can divide its class members to mutable and immutable types.
See some more details on the topic typescript clone array here:
ES6 Way to Clone an Array | SamanthaMing.com
When we need to copy an array, we often times used slice. But with ES6, you can also use the spread operator to duplicate an array. Pretty nifty, right.
How to clone an array in JavaScript – freeCodeCamp
How to clone an array in JavaScript · 1. Spread Operator (Shallow copy). Ever since ES6 dropped, this has been the most popular method. · 2. Good …
Cloning an Object in TypeScript | Delft Stack
Every element must be copied recursively to copy nested objects. In the case of arrays, the spread operator works in the case of primitive data …
How to clone Map with Array value in TypeScript – gists · GitHub
Example: How to clone Map with Array value in TypeScript – clone-map.ts.
How do you assign one array to another?
- Ensure that the two arrays have the same rank (number of dimensions) and compatible element data types.
- Use a standard assignment statement to assign the source array to the destination array. Do not follow either array name with parentheses.
How do you copy an array using the spread Operator?
The spread operator in ES6 is used to clone an array, whereas slice() method in JavaScript is an older way that provide 0 as the first argument. These methods create a new, independent array and copy all the elements of oldArray to the new one i.e. both these methods do a shallow copy of the original array.
How do you duplicate an object in JavaScript?
- Use the spread ( … ) syntax.
- Use the Object. assign() method.
- Use the JSON. stringify() and JSON. parse() methods.
Does spread Operator deep copy?
The spread operator makes deep copies of data if the data is not nested. When you have nested data in an array or object the spread operator will create a deep copy of the top most data and a shallow copy of the nested data.
What is a shallow copy JavaScript?
Shallow copy is a bit-wise copy of an object. A new object is created that has an exact copy of the values in the original object. If any of the fields of the object are references to other objects, just the reference addresses are copied i.e., only the memory address is copied.
Copying Arrays (deep and shallow) – Beau teaches JavaScript
Images related to the topicCopying Arrays (deep and shallow) – Beau teaches JavaScript
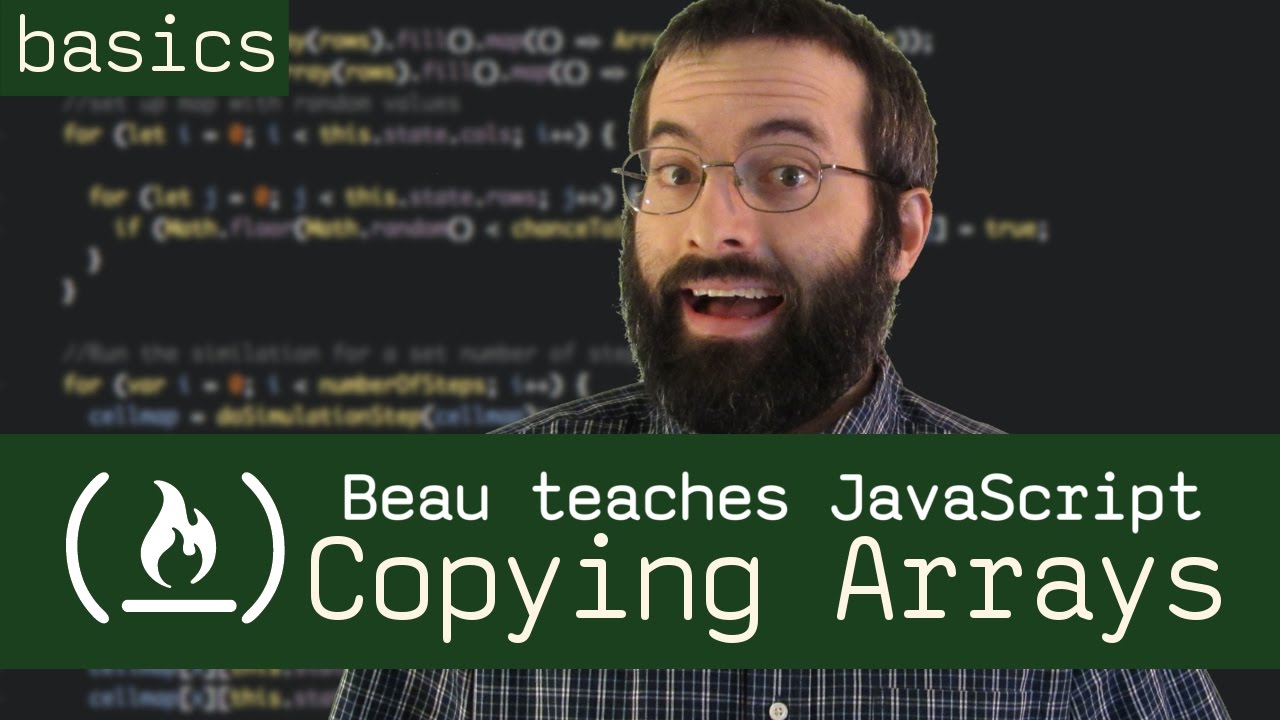
Which function is used to copy all the values from array to variable?
concat() techniques can be used to deep copy arrays with literal values (boolean, number, and string) only; where the Spread operator [… myArray ] has the best performance.
How do you move an object from one array to another?
- function arrMove(arr, oldIndex,
- if (newIndex >= arr. length) {
- let i = newIndex – arr. length.
- while (i–) {
- arr. push(undefined);
- arr. splice(newIndex, 0, arr. splice.
- return arr;
What is a shallow copy?
A shallow copy of an object is a copy whose properties share the same references (point to the same underlying values) as those of the source object from which the copy was made.
How can we copy object without mutating?
- You can use Object.assign : const a = { x: “Hi”, y: “Test” } const b = Object. assign({}, a, { x: “Bye” }); console. …
- Or you can use the object spread operator. The spread operator allows you to “assign” all properties of one object to another.
Is object assign deep copy?
Object. assign does not copy prototype properties and methods. This method does not create a deep copy of Source Object, it makes a shallow copy of the data. For the properties containing reference or complex data, the reference is copied to the destination object, instead of creating a separate object.
What is deep copy of object?
A deep copy of an object is a copy whose properties do not share the same references (point to the same underlying values) as those of the source object from which the copy was made.
How do I map one array to another array?
- Declare an array of any type, initialize an object array data.
- Declare a new result array of any type which is going to assign with part of the original array.
- Iterate an array using the forEach loop.
- add each item to a new result with the required fields.
- Finally, a new array is returned.
How do I merge two arrays in TypeScript?
The Array. concat() is an inbuilt TypeScript function which is used to merge two or more arrays together. Parameter: This method accepts a single parameter multiple time as mentioned above and described below: valueN : These parameters are arrays and/or values to concatenate.
How do you add an array to an array?
When you want to add an element to the end of your array, use push(). If you need to add an element to the beginning of your array, try unshift(). And you can add arrays together using concat().
Is ArrayList clone a deep copy?
ArrayList clone() method is used to create a shallow copy of the list.
TypeScript Part9- Arrays in TypeScript
Images related to the topicTypeScript Part9- Arrays in TypeScript

What is the difference between a shallow copy and a deep copy?
In Shallow copy, a copy of the original object is stored and only the reference address is finally copied. In Deep copy, the copy of the original object and the repetitive copies both are stored.
How do you copy an array to an ArrayList?
…
Conversion of Array To ArrayList in Java
- Using Arrays. asList() method – Pass the required array to this method and get a List object and pass it as a parameter to the constructor of the ArrayList class.
- Collections. …
- Iteration method – Create a new list.
Related searches to typescript clone array
- Remove item in array typescript
- Copy array object JavaScript
- remove item in array typescript
- deep copy array javascript
- Add array to array JavaScript
- Javascript copy array into another
- angular copy array without reference
- Copy array javascript
- copy array typescript
- Copy array TypeScript
- copy array javascript
- add array to array javascript
- typescript types list
- copy array object javascript
- javascript copy array into another
Information related to the topic typescript clone array
Here are the search results of the thread typescript clone array from Bing. You can read more if you want.
You have just come across an article on the topic typescript clone array. If you found this article useful, please share it. Thank you very much.