Are you looking for an answer to the topic “typescript copy array“? We answer all your questions at the website Chambazone.com in category: Blog sharing the story of making money online. You will find the answer right below.
Keep Reading
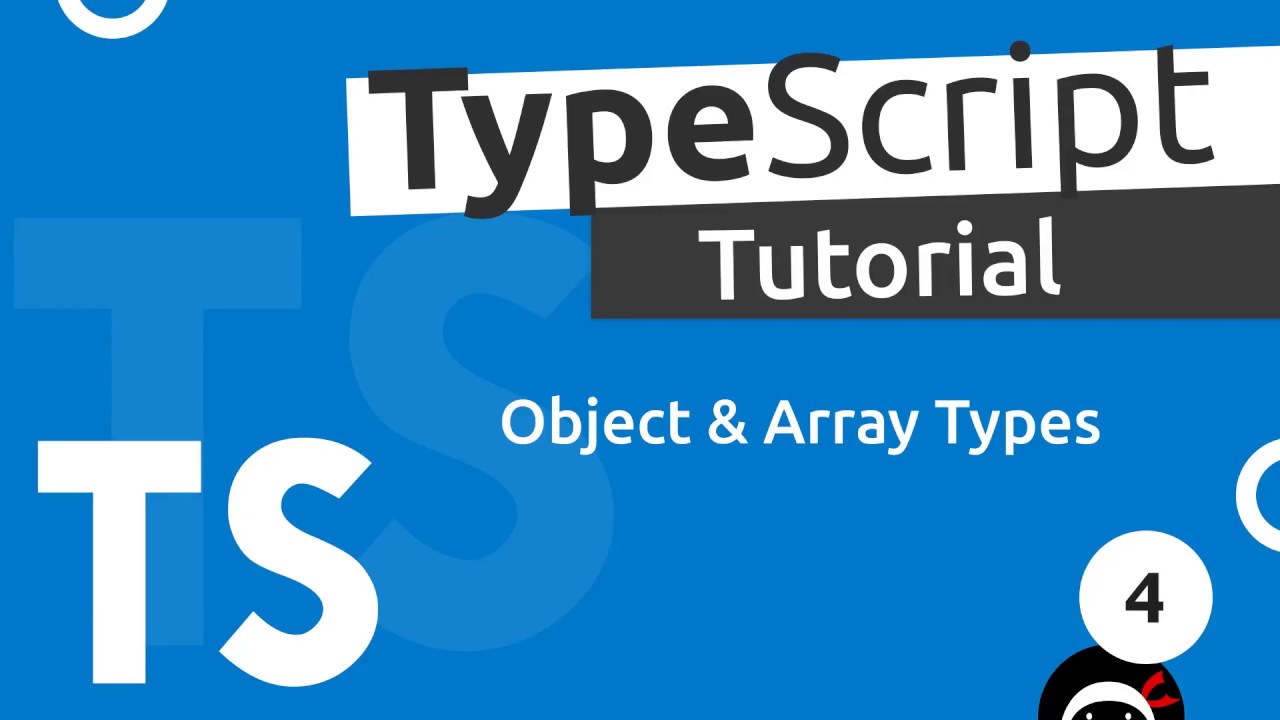
How do I copy one array to another in TypeScript?
- var ar = [“apple”,”banana”,”canaple”];
- var bar = Array. from(ar);
- alert(bar[1]); // alerts ‘banana’
How do you duplicate an array?
- You can use a for loop and copy elements of one to another one by one.
- Use the clone method to clone an array.
- Use arraycopy() method of System class.
- Use copyOf() or copyOfRange() methods of Arrays class.
TypeScript Tutorial #4 – Objects Arrays
Images related to the topicTypeScript Tutorial #4 – Objects Arrays
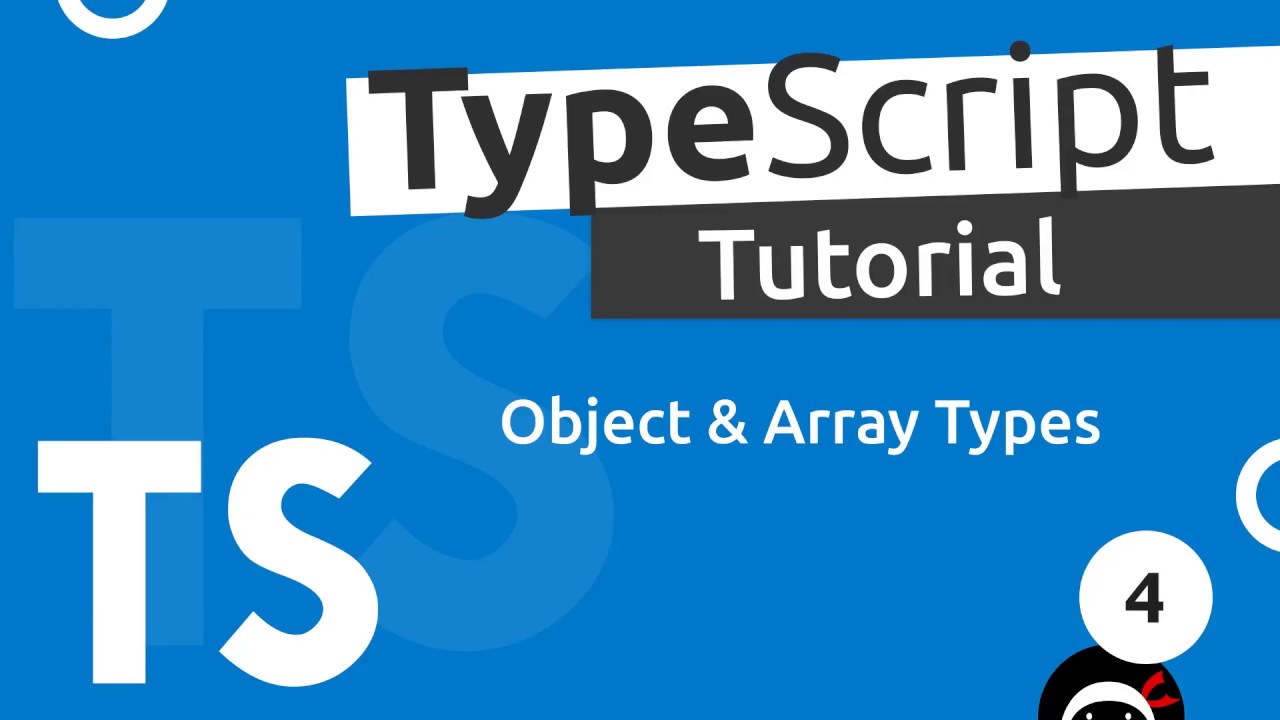
How do you copy an array in JavaScript?
Because arrays in JS are reference values, so when you try to copy it using the = it will only copy the reference to the original array and not the value of the array. To create a real copy of an array, you need to copy over the value of the array under a new value variable.
How do I copy values in TypeScript?
Use the Object. assign() Method to Clone an Object in TypeScript. The Object. assign() works similarly as in the case of the spread operator and can be used to clone simple objects, but it fails in the case of nested objects.
How do I push one array into another array?
- Javascript arrays concat method. let array1 = [1, 2]; let array2 = [3, 4]; let newArray = array1. …
- How to Copy Array Items into Another Array. function pushArray(arr, arr2) { arr. push. …
- Javascript arrays spread syntax. let array1 = [1, 2, 3]; let array2 = [4, 5]; array1.
How do you assign an array to another array?
- Ensure that the two arrays have the same rank (number of dimensions) and compatible element data types.
- Use a standard assignment statement to assign the source array to the destination array. Do not follow either array name with parentheses.
How do I make a deep copy of an ArrayList?
To create a true deep copy of ArrayList, we should create a new ArrayList and copy all the cloned elements to new ArrayList one by one and we should also clone Student object properly. To create deep copy of Student class, we can divide its class members to mutable and immutable types.
See some more details on the topic typescript copy array here:
ES6 Way to Clone an Array | SamanthaMing.com
When we need to copy an array, we often times used slice. But with ES6, you can also use the spread operator to duplicate an array. Pretty nifty, right.
Array.prototype.slice() – JavaScript – MDN Web Docs
The slice() method returns a shallow copy of a portion of an array into a new array object selected from start to end (end not included) …
How to clone an array in JavaScript – freeCodeCamp
Note: This doesn’t safely copy multi-dimensional arrays. Array/object values are copied by reference instead of by value. This is fine
How to copy objects in JavaScript: A complete guide
Here’s an example of shallow copy in TypeScript. … So, deepArray iterates over the provided array, calling deep for every value in it.
Does spread Operator deep copy?
The spread operator makes deep copies of data if the data is not nested. When you have nested data in an array or object the spread operator will create a deep copy of the top most data and a shallow copy of the nested data.
What is a shallow copy?
A shallow copy of an object is a copy whose properties share the same references (point to the same underlying values) as those of the source object from which the copy was made.
What are the 3 ways to copy an array?
- Using Modern ES6 Spread Operator. This is the modern method to clone an array in Javascript. …
- Using Slice. This is yet another popular way to copy an array in Javascript. …
- Using Concat. This method is another popular way to copy an array in Javascript.
Copying Arrays (deep and shallow) – Beau teaches JavaScript
Images related to the topicCopying Arrays (deep and shallow) – Beau teaches JavaScript
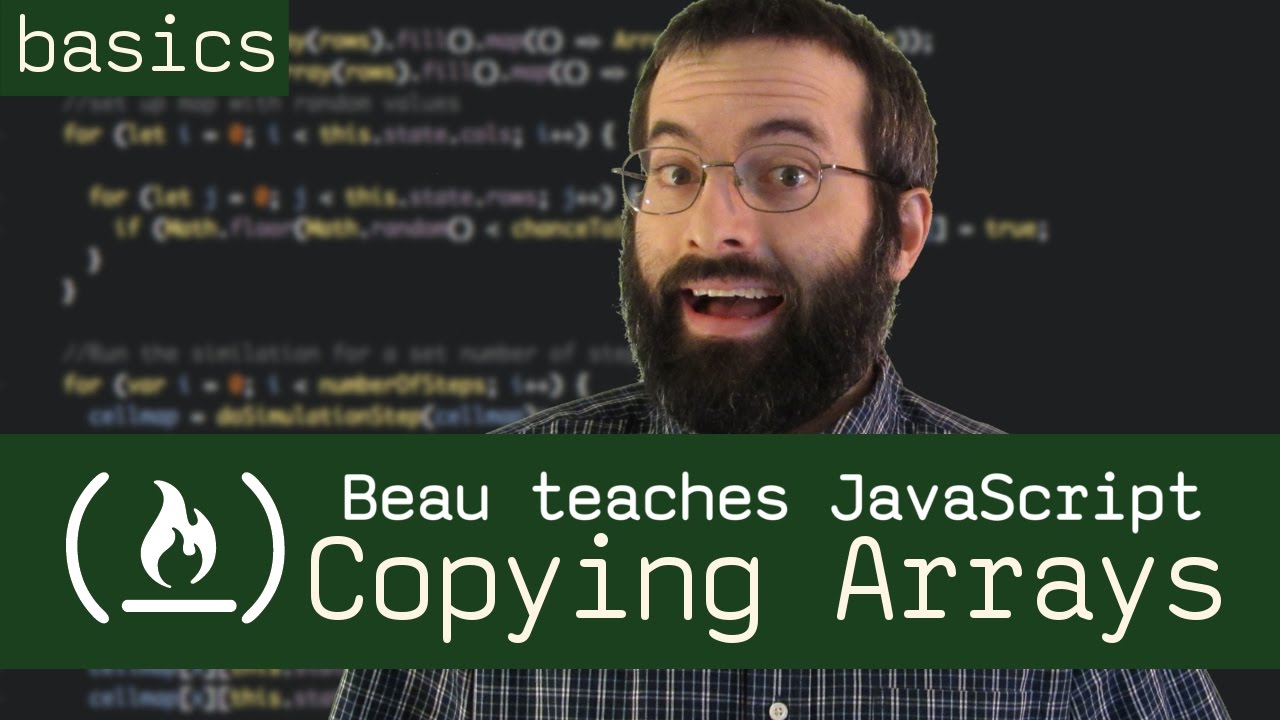
How many ways can you copy an array in JavaScript?
There are at least 6 (!) ways to clone an array: loop. slice.
How do you copy an object in JavaScript?
- Use the spread ( … ) syntax.
- Use the Object. assign() method.
- Use the JSON. stringify() and JSON. parse() methods.
How do you make a deep copy of an object in TypeScript?
- Use the JSON. stringify() method to stringify the object.
- Use the JSON. parse() method to parse the string back to an object.
- Use a type assertion to type the copied object.
What is the difference between a shallow copy and a deep copy?
In Shallow copy, a copy of the original object is stored and only the reference address is finally copied. In Deep copy, the copy of the original object and the repetitive copies both are stored.
What is a shallow copy JavaScript?
Shallow copy is a bit-wise copy of an object. A new object is created that has an exact copy of the values in the original object. If any of the fields of the object are references to other objects, just the reference addresses are copied i.e., only the memory address is copied.
How do you push an array into an object?
In order to push an array into the object in JavaScript, we need to utilize the push() function. With the help of Array push function this task is so much easy to achieve. push() function: The array push() function adds one or more values to the end of the array and returns the new length.
How do I copy one array to another pointer?
Logic to copy one array to another array using pointers
Declare another array say dest_array to store copy of source_array . Declare a pointer to source_array say *source_ptr = source_array and one more pointer to dest_array say *dest_ptr = dest_array .
Can an assignment operator copy one array to another?
Copy Array Using Assignment Operator
The assignment operator is used to assign a value to an array. By using the assignment operator, we can assign the contents of an existing array to a new variable, which will create a copy of our existing array.
Is ArrayList clone a deep copy?
ArrayList clone() method is used to create a shallow copy of the list.
TypeScript Part9- Arrays in TypeScript
Images related to the topicTypeScript Part9- Arrays in TypeScript

How do you copy an array to an ArrayList?
…
Conversion of Array To ArrayList in Java
- Using Arrays. asList() method – Pass the required array to this method and get a List object and pass it as a parameter to the constructor of the ArrayList class.
- Collections. …
- Iteration method – Create a new list.
Does ArrayList have a copy constructor?
Using a Copy Constructor: Using the ArrayList constructor in Java, a new list can be initialized with the elements from another collection. Syntax: ArrayList cloned = new ArrayList(collection c); where c is the collection containing elements to be added to this list.
Related searches to typescript copy array
- typescript copy part of array
- typescript copy array deep
- typescript copy array without reference
- typescript copy array spread
- typescript copy array of objects
- javascript copy array of objects without reference
- typescript types list
- typescript spread operator copy array
- deep copy array javascript
- typescript deep copy array
- typescript array remove duplicate
- angular typescript copy array
- typescript copy array of objects without reference
- typescript clone object
- typescript copy array elements
- typescript copy array splice
- javascript copy array
- typescript copy array slice
- typescript shallow copy array
Information related to the topic typescript copy array
Here are the search results of the thread typescript copy array from Bing. You can read more if you want.
You have just come across an article on the topic typescript copy array. If you found this article useful, please share it. Thank you very much.