Are you looking for an answer to the topic “typescript object.keys keyof“? We answer all your questions at the website Chambazone.com in category: Blog sharing the story of making money online. You will find the answer right below.
The TypeScript handbook documentation says: The keyof operator takes an object type and produces a string or numeric literal union of its keys. A simple usage is shown below. We apply the keyof operator to the Staff type, and we get a staffKeys type in return, which represents all the property names.The Object. keys() method returns an array of a given object’s own enumerable property names, iterated in the same order that a normal loop would.Use let k: keyof T and a for-in loop to iterate objects when you know exactly what the keys will be. Be aware that any objects your function receives as parameters might have additional keys. Use Object. entries to iterate over the keys and values of any object.
- Use the Object. keys() method to get an array of the object’s keys.
- Type the array to be an array of the object’s keys.
- Use the find() method to get the key by its value.
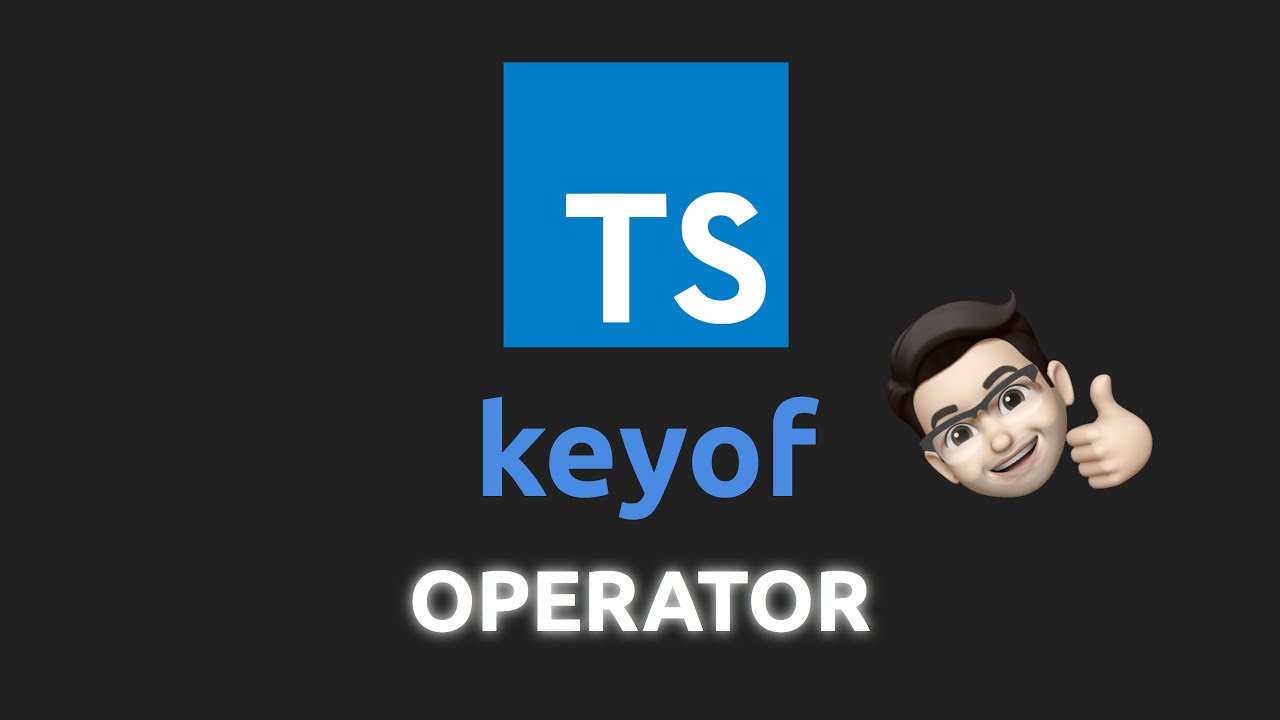
How do you get the keys of an object in TypeScript?
- Use the Object. keys() method to get an array of the object’s keys.
- Type the array to be an array of the object’s keys.
- Use the find() method to get the key by its value.
What does object keys do in TypeScript?
The Object. keys() method returns an array of a given object’s own enumerable property names, iterated in the same order that a normal loop would.
What does KEYOF mean in TypeScript (safety for dynamic JavaScript)
Images related to the topicWhat does KEYOF mean in TypeScript (safety for dynamic JavaScript)
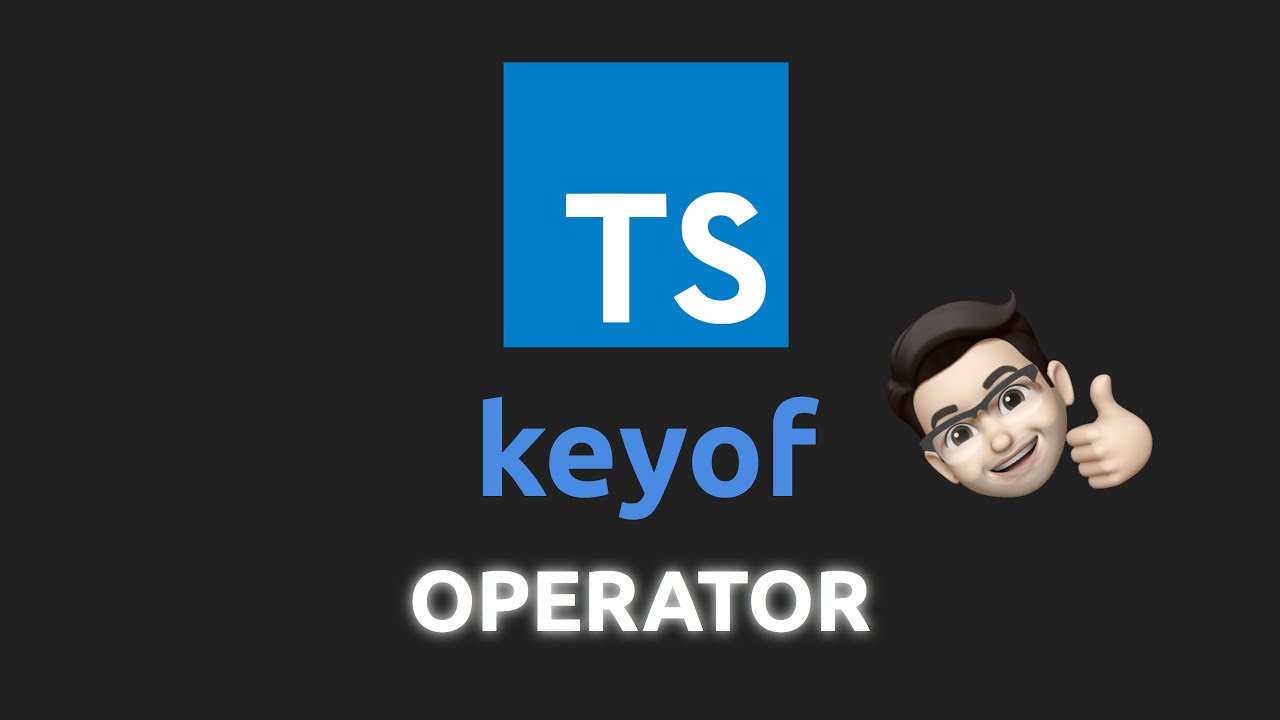
How do I iterate an object in TypeScript?
Use let k: keyof T and a for-in loop to iterate objects when you know exactly what the keys will be. Be aware that any objects your function receives as parameters might have additional keys. Use Object. entries to iterate over the keys and values of any object.
How do I get the key of an interface?
To get the union of the keys of a TypeScript interface as array of strings, we can use the keyof keyword. interface Person { name: string; age: number; location: string; } type Keys = keyof Person; to create the Keys type by setting it to keyof Person .
How do you find the key of an object?
- The Object.keys() method returns an array of strings containing all of the object’s keys, sorted by order of appearance:
- The Object.values() method returns an array of strings containing all of the object’s field values, sorted by order of appearance:
- The Object.
How do I find the key name of an object?
- First take the JavaScript Object in a variable.
- Use object. keys(objectName) method to get access to all the keys of object.
- Now, we can use indexing like Object. keys(objectName)[0] to get the key of first element of object.
What does Keyof do in TypeScript?
keyof takes an object type and returns a type that accepts any of the object’s keys.
See some more details on the topic typescript object.keys keyof here:
Documentation – Keyof Type Operator – TypeScript
The keyof type operator. The keyof operator takes an object type and produces a string or numeric literal union of its keys.
How keyof object operator works in typescript? – eduCBA
In typescript, keyof is defined as indexed type query operator for any object type this keyof the object type would be the union of properties of object names …
Create a Type from an object’s Keys in TypeScript | bobbyhadz
Use the keyof typeof syntax to create a type from an object’s keys, e.g. type Keys = keyof typeof person . The keyof typeof syntax returns a …
TypeScript explained in JavaScript: keyof | Spencer Miskoviak
This is where the keyof operator comes in. It conceptually behaves identical to the Object.keys method, but is a type instead of a literal value …
What is Keyof in JS?
The keyof operator takes an object type and produces a string or numeric literal union of its keys. The following type P is the same type as “x” | “y”: type Point = { x : number; y : number };
Are object keys ordered?
With arrays and other iterables (like strings, which allow you to iterate by character), you can use the for…of loop to access each item. With objects, you have to use the for…in loop instead. Since objects don’t have ordered object keys, you can’t use for…of .
How do you iterate over object properties?
- const items = { ‘first’: new Date(), ‘second’: 2, ‘third’: ‘test’ }
- items. map(item => {})
- items. forEach(item => {})
- for (const item of items) {}
- for (const item in items) { console. log(item) }
- Object. entries(items). map(item => { console. log(item) }) Object.
How do I use a key value pair in TypeScript?
Typescript Key Value Pair Working
A key-value pair is based on the structure of Key and value i.e the first step is to store the key-value and then associate the value of it in a Value tag. This is done with the help of API where we have the methods set and get a key to store the key.
Typescript’s keyof explained
Images related to the topicTypescript’s keyof explained

What line of code is used to iterate through all properties of an object?
The for…in statement iterates over all enumerable properties of an object that are keyed by strings (ignoring ones keyed by Symbols), including inherited enumerable properties.
What is difference between interface and type in typescript?
The typescript type supports only the data types and not the use of an object. The typescript interface supports the use of the object. Type keyword when used for declaring two different types where the variable names declared are the same then the typescript compiler will throw an error.
How can I get key and value from JSON object in typescript?
- myObject = {
- “key”: “value”
- }
-
- Object. keys(myObject); // get array of keys.
How do you access the key of an array of objects?
keys() returns an array of object’s keys, Object. values() returns an array of object’s values, and Object. entries() returns an array of object’s keys and corresponding values in a format [key, value] .
How do I print an object key?
- const object1 = {
- a: ‘somestring’,
- b: 42,
- c: false.
- };
-
- console. log(Object. keys(object1));
- // expected output: Array [“a”, “b”, “c”]
How do you access the properties of an object?
- Dot property accessor: object. property.
- Square brackets property access: object[‘property’]
- Object destructuring: const { property } = object.
How get key from value in HashMap?
Get keys from value in HashMap
To find all the keys that map to a certain value, we can loop the entrySet() and Objects. equals to compare the value and get the key. The common mistake is use the entry. getValue().
How do you find the value of an array of objects?
- Using Array. prototype. find() function. …
- Using Array. prototype. findIndex() function. …
- Using Array. prototype. forEach() function. …
- Using Array. prototype. …
- Using jQuery. The jQuery’s $. …
- Using Lodash/Underscore Library. The Underscore and Lodash library have the _.
Is Keyof TypeScript?
In the TypeScript world, the equivalent concept is the keyof operator. Although they are similar, keyof only works on the type level and returns a literal union type, while Object. keys returns values.
Typescript: keyof typeof
Images related to the topicTypescript: keyof typeof

What is a mapped object type?
A mapped type is a generic type which uses a union of PropertyKey s (frequently created via a keyof ) to iterate through keys to create a type: type OptionsFlags < Type > = { [ Property in keyof Type ]: boolean; };
What is infer in TypeScript?
Using infer in TypeScript
The infer keyword compliments conditional types and cannot be used outside an extends clause. Infer allows us to define a variable within our constraint to be referenced or returned.
Related searches to typescript object.keys keyof
- typescript get object by key
- typescript keyof
- key in typescript
- typescript object.keys keyof
- typescript get list of keys
- typescript object keys map
- typescript object key type enum
- typescript define object key
- typescript keyof array
- typescript list of keys
- typescript valueof
- typescript reduce object keys
- keyof interface typescript
- cannot find name keyof
Information related to the topic typescript object.keys keyof
Here are the search results of the thread typescript object.keys keyof from Bing. You can read more if you want.
You have just come across an article on the topic typescript object.keys keyof. If you found this article useful, please share it. Thank you very much.