Are you looking for an answer to the topic “typescript omit“? We answer all your questions at the website Chambazone.com in category: Blog sharing the story of making money online. You will find the answer right below.
Keep Reading
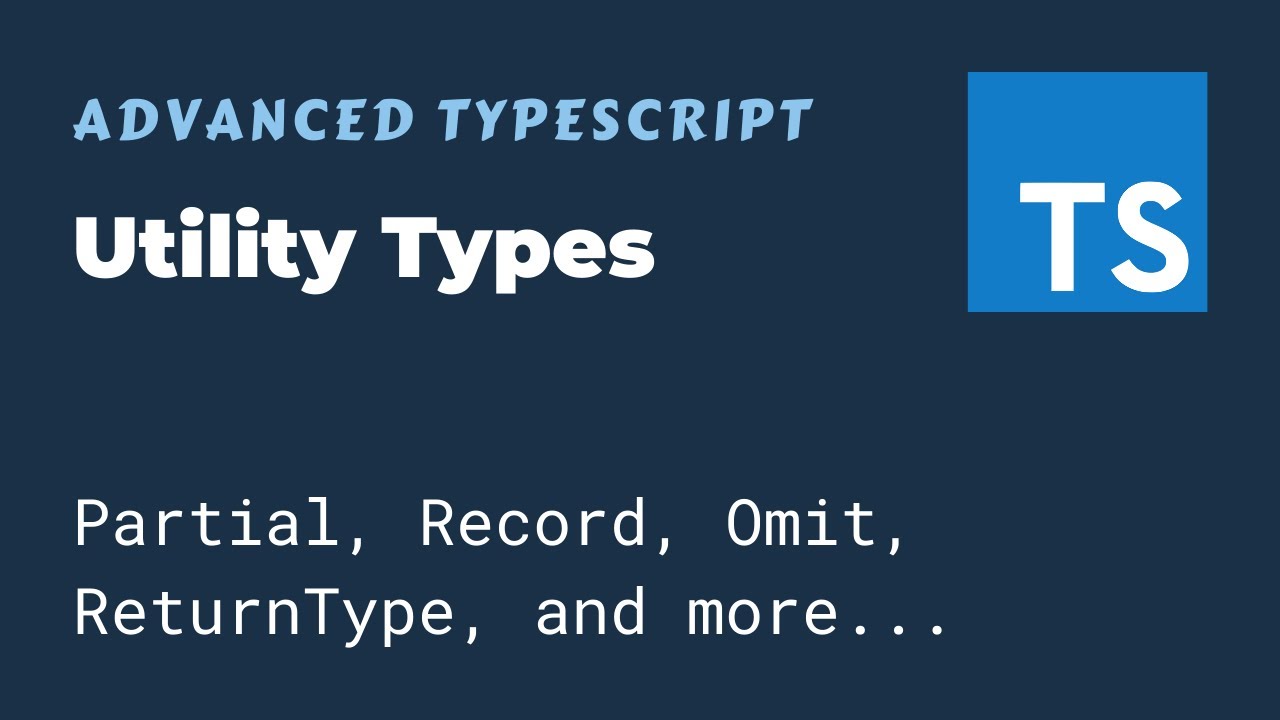
What is TypeScript omit?
The TypeScript Omit utility type
Like the Pick type, the Omit can be used to modify an existing interface or type. However, this one works the other way around. It will remove the fields you defined. We want to remove the id field from our user object when we want to create a user.
How do you omit a property in TypeScript?
Use the Omit utility type to exclude a property from a type, e.g. type WithoutCountry = Omit<Person, ‘country’> . The Omit utility type constructs a new type by removing the specified keys from the existing type.
Utility Types – Advanced TypeScript
Images related to the topicUtility Types – Advanced TypeScript
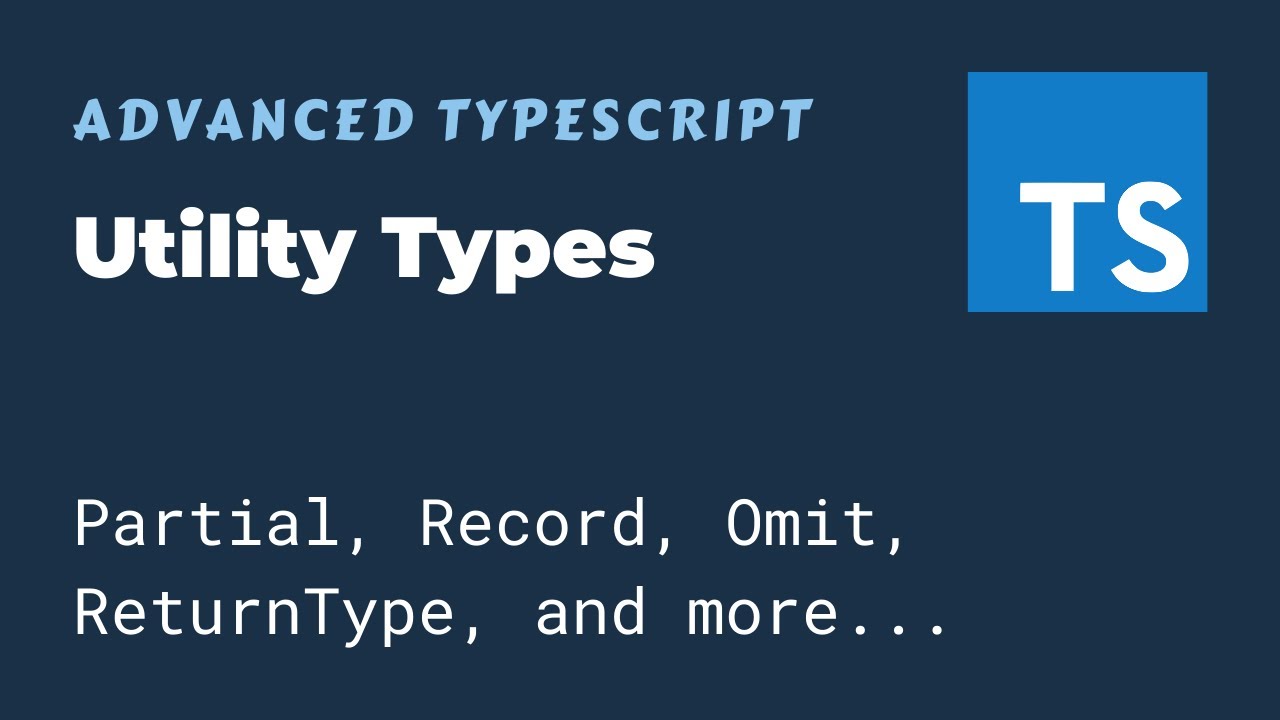
How does exclude work in TypeScript?
In TypeScript, the Exclude utility type lets us exclude certain members from an already defined union type. That means we can take an existing type, and remove items from it for specific situations.
How do you avoid in TypeScript?
any. ❌ Don’t use any as a type unless you are in the process of migrating a JavaScript project to TypeScript. The compiler effectively treats any as “please turn off type checking for this thing”. It is similar to putting an @ts-ignore comment around every usage of the variable.
What is ReturnType TypeScript?
ReturnType accepts a function and returns the return type that the function would return when being invoked. number in our getInt example. Taking this approach has one main advantage, we don’t need to keep the return types in sync with our function definition.
Why you should use TypeScript?
Typescript and NodeJS
Developers using JavaScript from other languages often complain about the lack of strong static characters, but that’s where TypeScript comes in to fill that gap. TypeScript is a superset of typed JavaScript (optional) that can help build and manage large-scale JavaScript projects.
How do I type a TypeScript function?
- let add: (x: number, y: number) => number; In this example: …
- add = function (x: number, y: number) { return x + y; }; …
- let add: (a: number, b: number) => number = function (x: number, y: number) { return x + y; }; …
- add = function (x: string, y: string): number { return x.concat(y).length; };
See some more details on the topic typescript omit here:
Documentation – Utility Types – TypeScript
Omit
The Omit Helper Type in TypeScript – Marius Schulz
In version 3.5, TypeScript added an Omit
TypeScript utility types: Pick and Omit – Daily Dev Tips
The TypeScript Omit utility type permalink … Like the Pick type, the Omit can be used to modify an existing interface or type. However, this one …
TypeScript | The Guide You Need to Learn the Omit Type
The omit utility type was introduced in TypeScript release 3.5 and it helps developers to generate new type definitions by omitting or excluding …
What is difference between interface and type in TypeScript?
The typescript type supports only the data types and not the use of an object. The typescript interface supports the use of the object. Type keyword when used for declaring two different types where the variable names declared are the same then the typescript compiler will throw an error.
What is the difference between interface vs type statements?
Interface declarations can exclusively represent the shape of an object-like data structures. Type alias declarations can create a name for all kind of types including primitives ( undefined , null , boolean , string and number ), union, and intersection types. In a way, this difference makes the type more flexible.
What is a Tsconfig file?
The tsconfig. json file allows you to specify the root level files and the compiler options that requires to compile a TypeScript project. The presence of this file in a directory specifies that the said directory is the TypeScript project root.
Is the operand of a delete operator must be optional?
The error “the operand of a ‘delete’ operator must be optional” occurs when we try to use the delete operator to delete a property that is marked as required. To solve the error use a question mark to mark the property as optional before using the delete operator.
Where can decorators be applied to?
Members of classes: These decorators are applied to the entire class. These functions are called with a single parameter which is to be decorated. This function is a constructor function. These decorators are not applied to each instance of the class, it only applies to the constructor function.
Typescript: Omit
Images related to the topicTypescript: Omit

How do I prevent TypeScript undefined?
To avoid undefined values when using or accessing the optional object properties, the basic idea is to check the property value using an if conditional statement or the optional chaining operator before accessing the object property in TypeScript.
Why you should not use any TypeScript?
Using any removes all type checking provided by TypeScript, which is one major reason for using TypeScript over JavaScript. By using any , you expose yourself to issues that are difficult to trace and debug, especially once the code is deployed in production.
What can I use instead of any in TypeScript?
unknown can usually be used instead
It is similar to any in that it can be “anything”, but has one huge difference: it can only be interacted with once we know the type (through something like a type guard or inference). In most cases, you can do the ol’ switcheroo and unknown will work fine where you once had any .
What does three dots mean in TypeScript?
The three dots are known as the spread operator from Typescript (also from ES7). The spread operator return all elements of an array. Like you would write each element separately: let myArr = [1, 2, 3]; return [1, 2, 3]; //is the same as: return […myArr];
How do you cast on TypeScript?
JavaScript doesn’t have a concept of type casting because variables have dynamic types. However, every variable in TypeScript has a type. Type castings allow you to convert a variable from one type to another. In TypeScript, you can use the as keyword or <> operator for type castings.
What is utility types in TypeScript?
TypeScript Utility Types. Paid Courses Website NEW Pro NEW. Dark mode. Dark code.
Is TypeScript slower than JavaScript?
TS type system is exceptionally rich and powerful, way more powerful than that of Java or Scala. This also means that the size of a code that infers types is huge. Unlike many other languages, TS is written on a slow scripting language — JavaScript.
Is TypeScript better than Python?
In terms of raw performance, Typescript is much faster than Python. When coding memory-intensive tasks in Python, e.g games, that utilize high-end 3D graphics, the CPU begins to take a hit and there is a significant drop in performance. Unlike Typescript, Python is not asynchronous at its core.
Is TypeScript better than JavaScript?
Advantages of using TypeScript over JavaScript
TypeScript always points out the compilation errors at the time of development (pre-compilation). Because of this getting runtime errors is less likely, whereas JavaScript is an interpreted language. TypeScript supports static/strong typing.
How do I declare optional parameters in TypeScript?
Use the parameter?: type syntax to make a parameter optional. Use the expression typeof(parameter) !== ‘undefined’ to check if the parameter has been initialized.
No BS TS #9 – Typescript Utility Types
Images related to the topicNo BS TS #9 – Typescript Utility Types
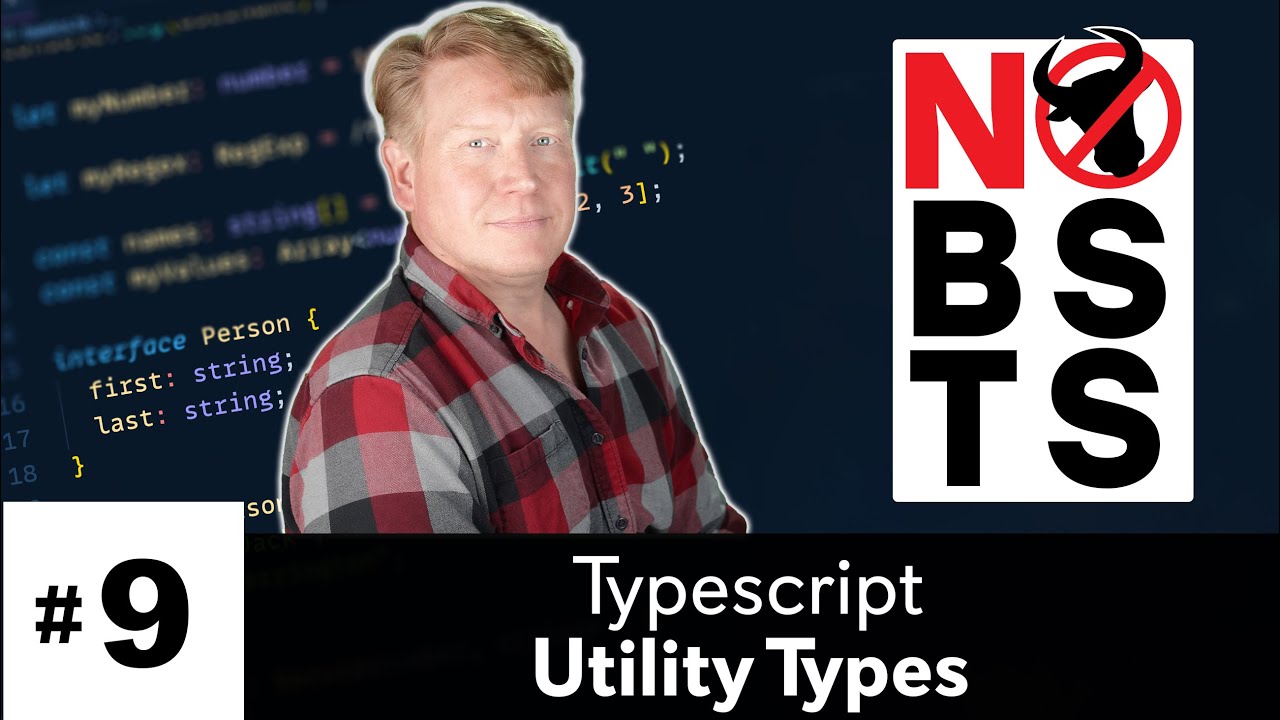
What is optional parameter function?
By definition, an Optional Parameter is a handy feature that enables programmers to pass less number of parameters to a function and assign a default value.
How do you pass a function as argument TypeScript?
Similar to JavaScript, to pass a function as a parameter in TypeScript, define a function expecting a parameter that will receive the callback function, then trigger the callback function inside the parent function.
Related searches to typescript omit
- typescript omit vs exclude
- typescript omit example
- typescript omit from union
- typescript pick omit
- typescript omit undefined
- typescript omit multiple
- typescript omit enum
- typescript omit nested
- typescript extends omit
- typescript interface extends omit
- typescript omit property
- typescript omit function
- typescript opposite of omit
- typescript pick
- typescript omit opposite
- typescript lodash omit
- typescript omit pick
- typescript omit interface
Information related to the topic typescript omit
Here are the search results of the thread typescript omit from Bing. You can read more if you want.
You have just come across an article on the topic typescript omit. If you found this article useful, please share it. Thank you very much.