Are you looking for an answer to the topic “typescript uuid“? We answer all your questions at the website Chambazone.com in category: Blog sharing the story of making money online. You will find the answer right below.
Keep Reading
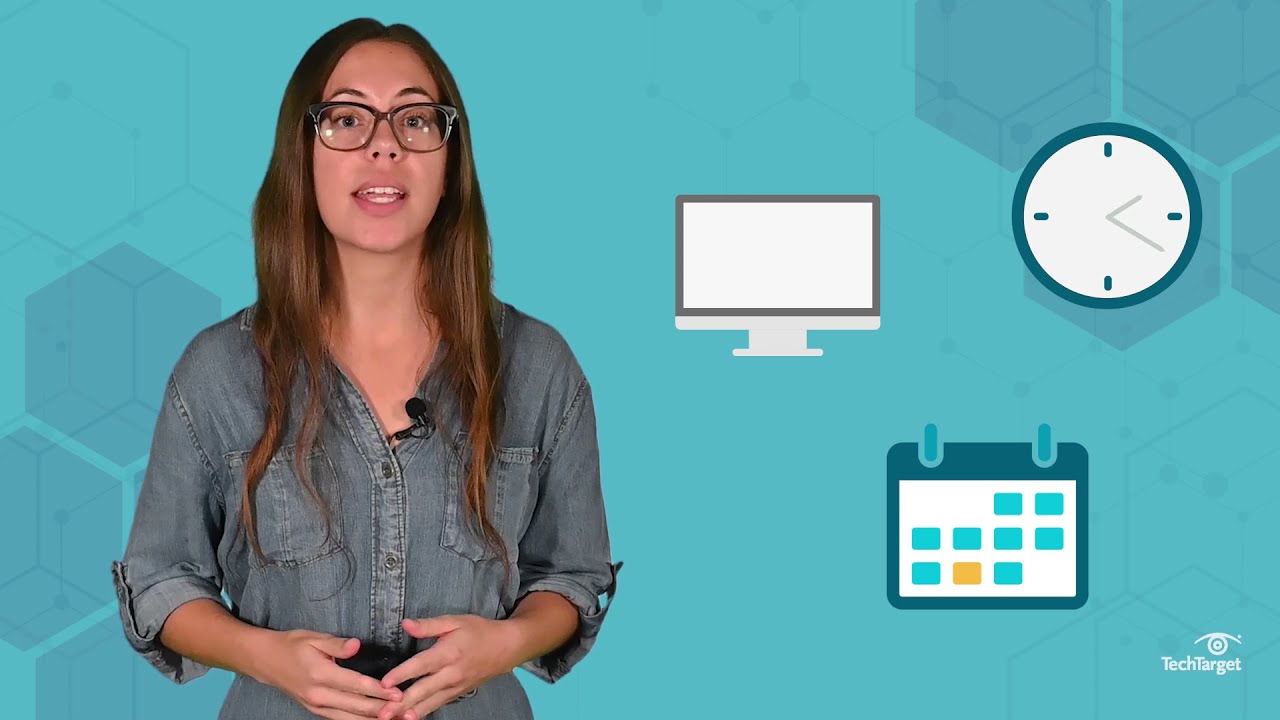
Is there a UUID type in TypeScript?
TypeScript program generates a Universally Unique Identifier or UUID by replacing the standard value with hexadecimal characters and generating random numbers cryptographically and displaying the generated UUID as the output of the screen. In the above program, ([1e7]+-1e3+-4e3+-8e3+-1e11).
How do you generate a random UUID in TypeScript?
- function uuid() {
- return ‘xxxxxxxx-xxxx-4xxx-yxxx-xxxxxxxxxxxx’. replace(/[xy]/g, function(c) {
- var r = Math. random() * 16 | 0, v = c == ‘x’ ? r : (r & 0x3 | 0x8);
- return v. toString(16);
- });
- }
-
- var userID=uuid();//something like: “ec0c22fa-f909-48da-92cb-db17ecdb91c5”
What is a UUID? UUID vs. GUID
Images related to the topicWhat is a UUID? UUID vs. GUID
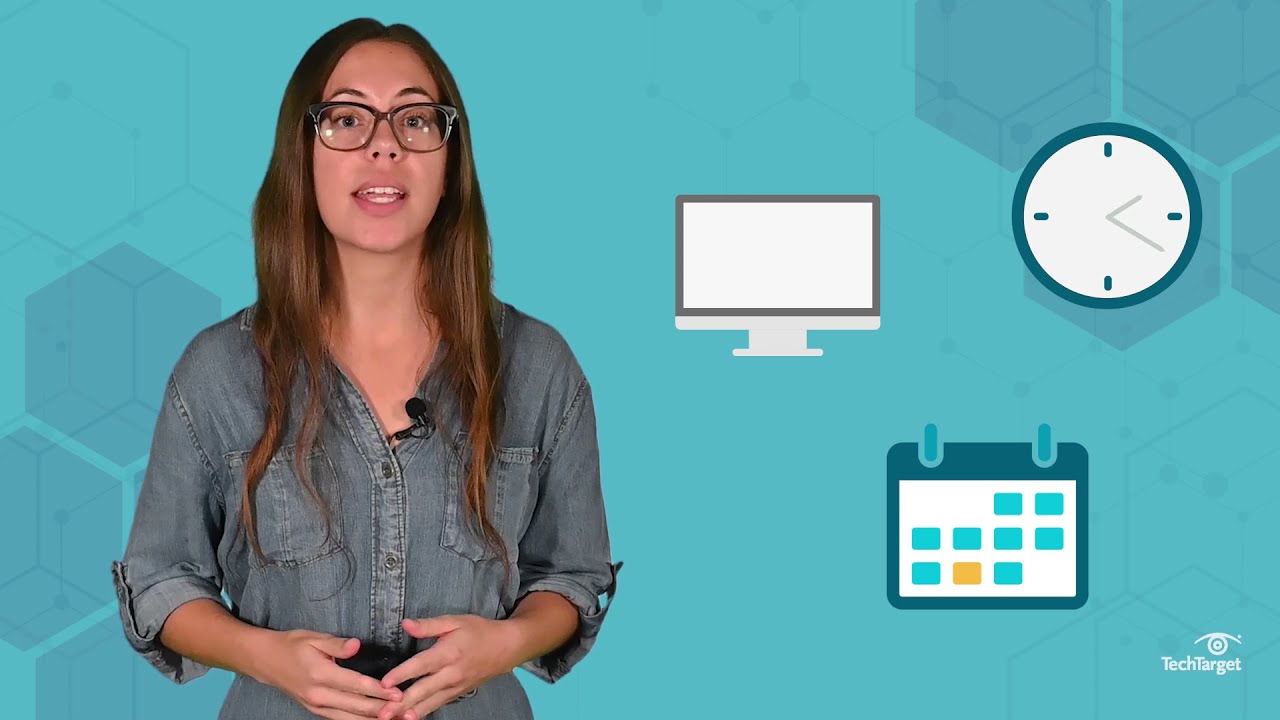
What is UUID in angular?
An AngularJS wrapper for a rigorous implementation of RFC4122 (v1 and v4) UUIDs.
Is a GUID a UUID?
The GUID designation is an industry standard defined by Microsoft to provide a reference number which is unique in any context. UUID is a term that stands for Universal Unique Identifier. Similarly, GUID stands for Globally Unique Identifier. So basically, two terms for the same thing.
Are UUID always unique?
No, a UUID can’t be guaranteed to be unique. A UUID is just a 128-bit random number. When my computer generates a UUID, there’s no practical way it can prevent your computer or any other device in the universe from generating that same UUID at some time in the future.
How do you represent a GUID in TypeScript?
Guids are usually represented as strings in Javascript, so the simplest way to represent the GUID is as a string. Usually when serialization to JSON occurs it is represented as a string, so using a string will ensure compatibility with data from the server.
How do I generate a random number in TypeScript?
- // Between any two numbers.
- Math. floor(Math. random() * (max – min + 1)) + min;
- // Between 0 and max.
- Math. floor(Math. random() * (max + 1));
- // Between 1 and max.
- Math. floor(Math. random() * max) + 1;
See some more details on the topic typescript uuid here:
Generate a UUID in TypeScript
The JavaScript library we recommend for generating UUIDs in TypeScript is called (unsurprisingly), uuid . It can generate version 1, 3, 4 and 5 UUIDs.
A Complete Guide to TypeScript UUID – eduCBA
A Universal Unique Identifier is called UUID in TypeScript. They are also known as Globally Unique Identifiers represented as GUID and is a Microsoft …
How to generate uuid in TypeScript? – Poopcode
const myUUID = uuid(); // Method 2: manually write uuid function const uuid = () => “xxxxxxxx-xxxx-4xxx-yxxx-xxxxxxxxxxxx”.replace(/[xy]/g …
Use Uuid in TypeScript Nodejs App | Delft Stack
This tutorial illustrates the ways to use uuid in the TypeScript Nodejs App. This will provide complete coding examples of using the npm …
What does UUID look like?
Format. In its canonical textual representation, the 16 octets of a UUID are represented as 32 hexadecimal (base-16) digits, displayed in five groups separated by hyphens, in the form 8-4-4-4-12 for a total of 36 characters (32 hexadecimal characters and 4 hyphens). For example: 123e4567-e89b-12d3-a456-426614174000.
What is namespace in UUID?
The namespace is either a UUID in string representation or an identifier for internally pre-defined namespace UUIDs (currently known are “ns:DNS”, “ns:URL”, “ns:OID”, and “ns:X500”). The name is a string of arbitrary length. The namespace is whatever UUID you like.
What is UUID JavaScript?
According to Wikipedia – A universally unique identifier (UUID) is an identifier standard used in software construction. A UUID is simply a 128-bit value. The meaning of each bit is defined by any of several variants.
How does angular 8 generate random numbers?
AngularJs Generate Random Number Example
var randNumber = Math. random() * 1000; Multiply by number such as 1000, 10,000 to generate random number between the specified limit.
Could not find a declaration file for module react UUID?
The error “could not find declaration file for module uuid ” occurs when TypeScript cannot find the type declaration for a uuid-related module. To solve the error install the types for the module by running the command from the error message, e.g. npm install -D @types/uuid .
React Todo List App with Typescript – Part 2 – Props, States uuid
Images related to the topicReact Todo List App with Typescript – Part 2 – Props, States uuid

Should I use UUID as primary key?
Pros. Using UUID for a primary key brings the following advantages: UUID values are unique across tables, databases, and even servers that allow you to merge rows from different databases or distribute databases across servers. UUID values do not expose the information about your data so they are safer to use in a URL.
Why should we use UUID?
UUIDs are generally used for identifying information that needs to be unique within a system or network thereof. Their uniqueness and low probability in being repeated makes them useful for being associative keys in databases and identifiers for physical hardware within an organization.
How do I create a new UUID?
- Generate 16 random bytes (=128 bits)
- Adjust certain bits according to RFC 4122 section 4.4 as follows: …
- Encode the adjusted bytes as 32 hexadecimal digits.
- Add four hyphen “-” characters to obtain blocks of 8, 4, 4, 4 and 12 hex digits.
How many UUIDs are possible?
32 hexadecimals x log2(16) bits/hexadecimal = 128 bits in a UUID. In the version 4, variant 1 type of UUID, 6 bits are fixed and the remaining 122 bits are randomly generated, for a total of 2¹²² possible UUIDs.
Will we ever run out of UUIDs?
Absolutely. Even if only one GUID is generated per second, we’ll run out in a scant 9 quintillion years. That’s well before the heat death of the Universe.
How do I get 16 digit UUID?
- You can maintain some long counter (to ensure that the generated identifiers are unique)
- or generate a random long – which runs the risk of getting repeated values.
What is GUID code?
A GUID (globally unique identifier) is a 128-bit text string that represents an identification (ID). Organizations generate GUIDs when a unique reference number is needed to identify information on a computer or network. A GUID can be used to ID hardware, software, accounts, documents and other items.
What is an empty GUID?
Empty Guids
As the Guid structure cannot be set to null, a value must be used to represent an ID that is not set. This value, known as the empty GUID, consists only of zeroes. This is the same value that is generated by the parameter-less constructor.
How do I create a date in typescript?
- new Date(): It creates a new date object with the current date and time. …
- new Date(milliseconds): It creates a new date object as zero time plus milliseconds. …
- new Date(datestring): It creates a new date object from a date string.
How do you generate random numbers?
Computers can generate truly random numbers by observing some outside data, like mouse movements or fan noise, which is not predictable, and creating data from it. This is known as entropy. Other times, they generate “pseudorandom” numbers by using an algorithm so the results appear random, even though they aren’t.
Fullstack2021 | p69 | UUID | Start using a UUID instead of a Socket id
Images related to the topicFullstack2021 | p69 | UUID | Start using a UUID instead of a Socket id

How do you generate a random number in JavaScript?
Javascript creates pseudo-random numbers with the function Math. random() . This function takes no parameters and creates a random decimal number between 0 and 1. The returned value may be 0, but it will never be 1.
How do I convert a number to a string in TypeScript?
Use the String() object to convert a number to a string in TypeScript, e.g. const str = String(num) . When used as a function, the String object converts the passed in value to a primitive string and returns the result. Copied!
Related searches to typescript uuid
- typescript uuid4
- typescript uuid v4
- typescript uuid library
- node uuid
- typescript uuid validator
- typescript uuid interface
- uuid npm typescript
- node typescript uuid
- react native uuid
- typescript random uuid generator
- typescript import uuid
- typescript uuid type
- typescript uuid generator
- @types/uuid
- typescript generate uuid
- typescript validate uuid
- typescript uuid npm
- react typescript uuid
- typesuuid
- react uuid typescript
- npm uuid
- sequelize typescript uuid
- typescript uuid angular
- typescript uuid example
- typescript new uuid
- typescript short uuid
- angular typescript uuid
Information related to the topic typescript uuid
Here are the search results of the thread typescript uuid from Bing. You can read more if you want.
You have just come across an article on the topic typescript uuid. If you found this article useful, please share it. Thank you very much.