Are you looking for an answer to the topic “update datetime python“? We answer all your questions at the website Chambazone.com in category: Blog sharing the story of making money online. You will find the answer right below.
You can’t change a DateTime value – it’s immutable. However, you can change the variable to have a new value. The easiest way of doing that to change just the time is to create a TimeSpan with the relevant time, and use the DateTime.Python Datetime module comes built into Python, so there is no need to install it externally. Python Datetime module supplies classes to work with date and time. These classes provide a number of functions to deal with dates, times and time intervals.
- from datetime import datetime.
- date = datetime. strptime(’26 Sep 2012′, ‘%d %b %Y’)
- newdate = date. replace(hour=11, minute=59)
-
- import time.
- timestamp = 1547281745.
- datetime = time. strftime(‘%A, %Y-%m-%d %H:%M:%S’, time. localtime(timestamp))
- print(datetime)
- import datetime.
-
- today = datetime. datetime. now()
- date_time = today. strftime(“%m/%d/%Y, %H:%M:%S”)
- print(“date and time:”,date_time)
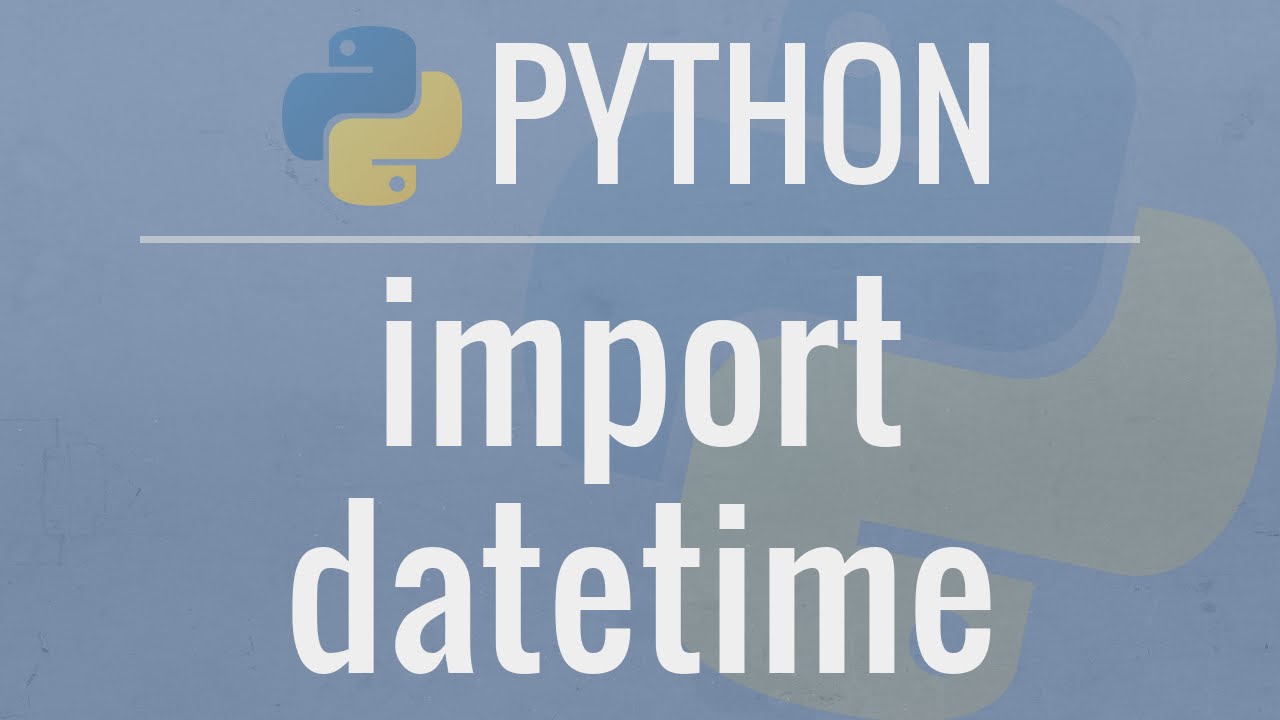
How do you edit datetime?
You can’t change a DateTime value – it’s immutable. However, you can change the variable to have a new value. The easiest way of doing that to change just the time is to create a TimeSpan with the relevant time, and use the DateTime.
How do I change the timestamp in Python?
- import time.
- timestamp = 1547281745.
- datetime = time. strftime(‘%A, %Y-%m-%d %H:%M:%S’, time. localtime(timestamp))
- print(datetime)
Python Tutorial: Datetime Module – How to work with Dates, Times, Timedeltas, and Timezones
Images related to the topicPython Tutorial: Datetime Module – How to work with Dates, Times, Timedeltas, and Timezones
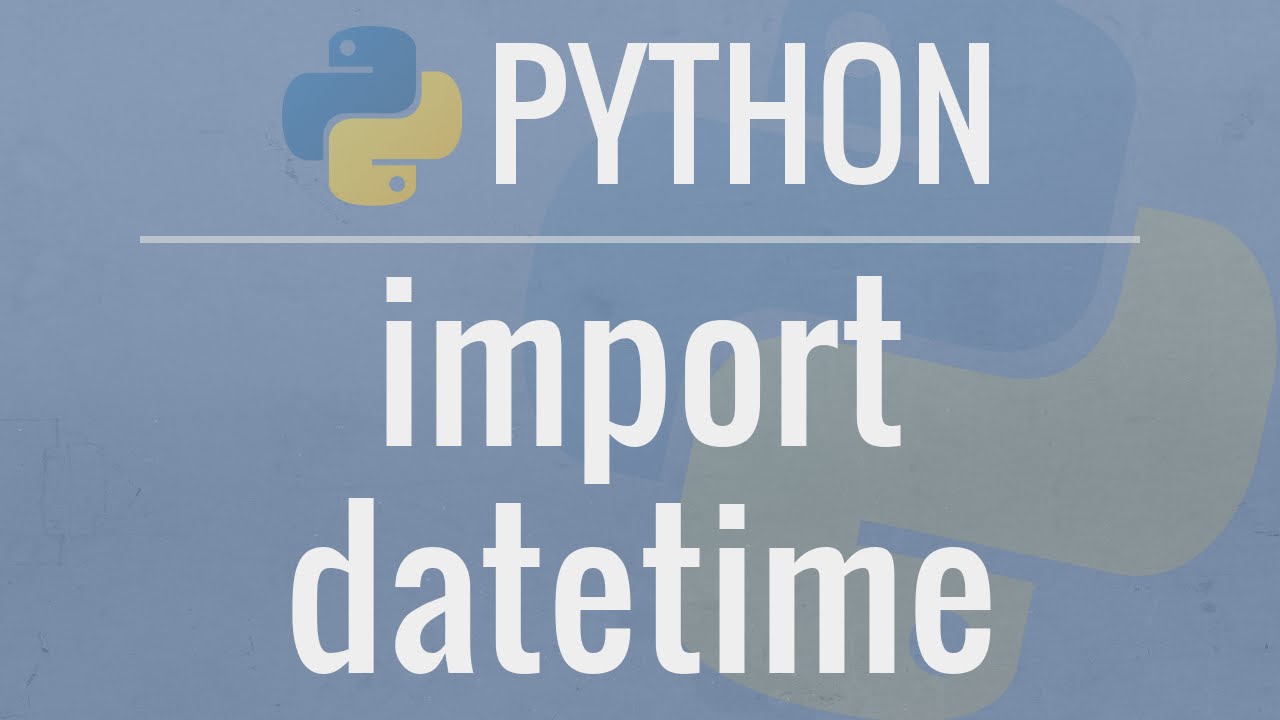
What package is datetime in Python?
Python Datetime module comes built into Python, so there is no need to install it externally. Python Datetime module supplies classes to work with date and time. These classes provide a number of functions to deal with dates, times and time intervals.
How do I append a date in Python?
- import datetime.
-
- today = datetime. datetime. now()
- date_time = today. strftime(“%m/%d/%Y, %H:%M:%S”)
- print(“date and time:”,date_time)
How do you change the time?
- Open your phone’s Clock app .
- Tap More. Settings. To pick your home time zone: Tap Home time zone. To automatically update your timezone: Tap Change date & time. Set time zone automatically. To update your timezone based on your location: Tap Change date & time Set time zone automatically.
How do I change the format of a datetime object in Python?
Use datetime. strftime(format) to convert a datetime object into a string as per the corresponding format . The format codes are standard directives for mentioning in which format you want to represent datetime. For example, the %d-%m-%Y %H:%M:%S codes convert date to dd-mm-yyyy hh:mm:ss format.
How do I change timestamp on pandas?
replace() function is used to replace the member values of the given Timestamp. The function implements datetime. replace, and it also handles nanoseconds.
See some more details on the topic update datetime python here:
How to modify datetime.datetime.hour in Python? – Stack …
Use the replace method to generate a new datetime object based on your existing one: tomorrow = tomorrow.replace(hour=12).
datetime — Basic date and time types — Python 3.10.4 …
datetime — Basic date and time types¶. Source code: Lib/datetime.py. The datetime module supplies classes for manipulating dates and times.
DateTime – PyPI
This package provides a DateTime data type, as known from Zope. Unless you need to communicate with Zope APIs, you’re probably better off using Python’s …
datetime – Date/time value manipulation – Python … – PyMOTW
Purpose: The datetime module includes functions and classes for doing date and time parsing, formatting, and arithmetic. Available In: 2.3 and later …
How do I use datetime Strptime in Python?
…
How strptime() works?
format code | meaning | example |
---|---|---|
%H | hour(24 hour clock) as a zero padded decimal number | 01, 23 |
%-H | hour(24 hour clock) as a decimal number | 1, 23 |
How do I get the current date in Python?
- from datetime import datetime # Current date time in local system print(datetime.now())
- print(datetime.date(datetime.now()))
- print(datetime.time(datetime.now()))
- pip install pytz.
How do I add time to a datetime?
- date_and_time = datetime. datetime(2020, 2, 19, 12, 0, 0)
- print(date_and_time)
- time_change = datetime. timedelta(hours=10)
- new_time = date_and_time + time_change.
- print(new_time)
Datetime Module (Dates and Times) || Python Tutorial || Learn Python Programming
Images related to the topicDatetime Module (Dates and Times) || Python Tutorial || Learn Python Programming
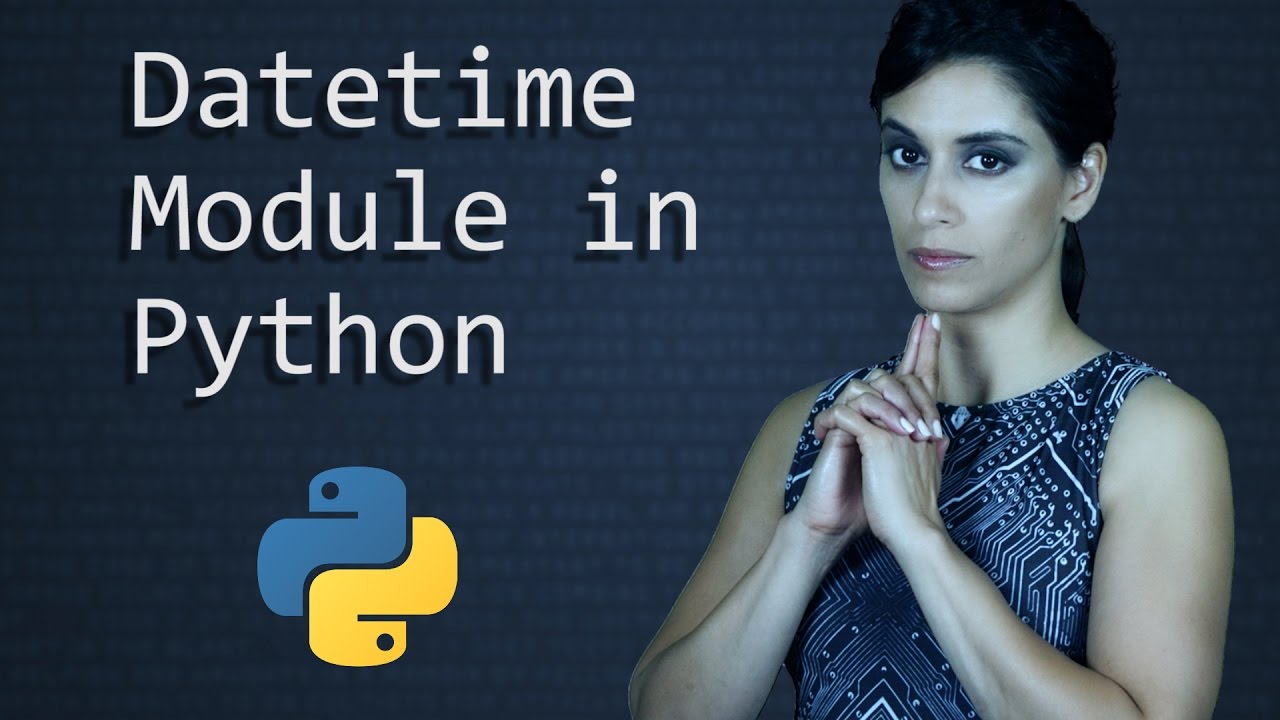
How do I increment a datetime in Python?
- date = datetime(2020, 2, 20)
- date += timedelta(days=1)
- print(date)
How do you add days to a datetime date?
Use timedelta in datetime Module to Add Days to the Current Date. The Python datetime has the timedelta method itself besides the timedelta from its submodule datetime . The timedelta() method takes the number of days to be added as its argument and returns them in date format.
How do I enable date and time change?
To change the date and time in Windows 10, open the “Settings” window. Then click the “Time & language” button in the middle of the screen to display time and language settings. Then click the “Date & time” category at the left side of this window to view date and time settings in the area to the right.
Why is my automatic date and time wrong?
Turn on Android’s automatic date/time setting. Do this through Settings > System > Date & time. Select the button next to Set time automatically to trigger it. If this is already turned on, turn it off, restart your phone, and then turn it back on.
How do I change the date format in a Dataframe in Python?
- # change the format to DD-MM-YYYY. df[‘Col’] = df[‘Col’].dt. …
- # covert to datetime. df[‘Birthday’] = pd. …
- # date in MM-DD-YYYY format. df[‘Birthday2’] = df[‘Birthday’].dt. …
- # date in DD-MM-YYYY format. df[‘Birthday3’] = df[‘Birthday’].dt. …
- # date in Month day, Year format.
How do I change data type in Python?
astype() method. We can pass any Python, Numpy or Pandas datatype to change all columns of a dataframe to that type, or we can pass a dictionary having column names as keys and datatype as values to change type of selected columns.
How does Python determine date format?
- date_string = ’12-25-2018′
- format = “%Y-%m-d”
- try:
- datetime. datetime. strptime(date_string, format)
- print(“This is the correct date string format.”)
- except ValueError:
- print(“This is the incorrect date string format. It should be YYYY-MM-DD”)
What is timestamp () Python?
Timestamp is the pandas equivalent of python’s Datetime and is interchangeable with it in most cases. It’s the type used for the entries that make up a DatetimeIndex, and other timeseries oriented data structures in pandas.
Ultimate Guide to Datetime! Python date and time objects for beginners
Images related to the topicUltimate Guide to Datetime! Python date and time objects for beginners
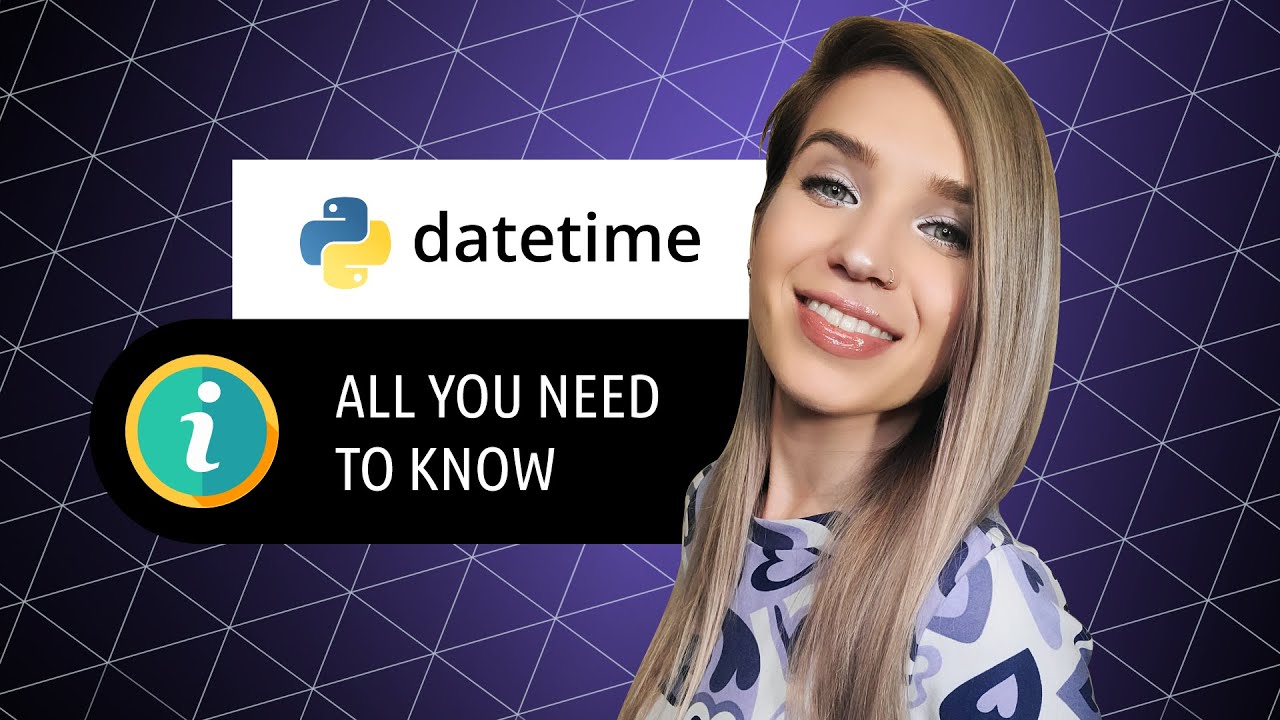
How do I remove timestamp from time in Python?
To remove the time from a datetime object in Python, convert the datetime to a date using date(). You can also use strftime() to create a string from a datetime object without the time.
How do I convert a column to a timestamp in Python?
- import pandas as pd.
-
- #create DataFrame.
- df = pd. DataFrame({‘stamps’: pd. date_range(start=’2020-01-01 12:00:00′,
- periods=6,
- freq=’H’),
- ‘sales’: [11, 14, 25, 31, 34, 35]})
-
Related searches to update datetime python
- python sql update datetime
- how to get day from datetime in python
- python datetime update hour
- python datetime set hour minute second to 0
- last_updated datetime python
- python mysql update datetime
- python datetime update timestamp
- change datetime format python
- python datetime update timezone
- python datetime set time to 0
- update time in datetime python
- python datetime update time
- datetime replace
- python datetime now update
- python datetime update month
- datetime change year python
- convert datetime to time python
- python django update datetime field
- datetime change hour python
- update value datetime python
Information related to the topic update datetime python
Here are the search results of the thread update datetime python from Bing. You can read more if you want.
You have just come across an article on the topic update datetime python. If you found this article useful, please share it. Thank you very much.