Are you looking for an answer to the topic “write dict to file“? We answer all your questions at the website Chambazone.com in category: Blog sharing the story of making money online. You will find the answer right below.
We can write dictionary to file by using json. dumps, for loop with dictionary items(), str(), keys() and pickle. dump() method.Easiest way is to open a csv file in ‘w’ mode with the help of open() function and write key value pair in comma separated form. The csv module contains DictWriter method that requires name of csv file to write and a list object containing field names.
- Import Json.
- Create A Dictionary in-order pass it into text file.
- Open file in write mode.
- Use json. dumps() for json string.
- dictionary_data = {“a”: 1, “b”: 2}
- a_file = open(“data.json”, “w”)
- json. dump(dictionary_data, a_file)
- a_file. close()
- a_file = open(“data.json”, “r”)
- output = a_file. read()
- print(output)
- a_file. close()
…
2. Pickle Module (Recommended)
- Importing the pickle module.
- Opening the file in write/append binary mode.
- Entering the data into the file using pickle module’s dump method.
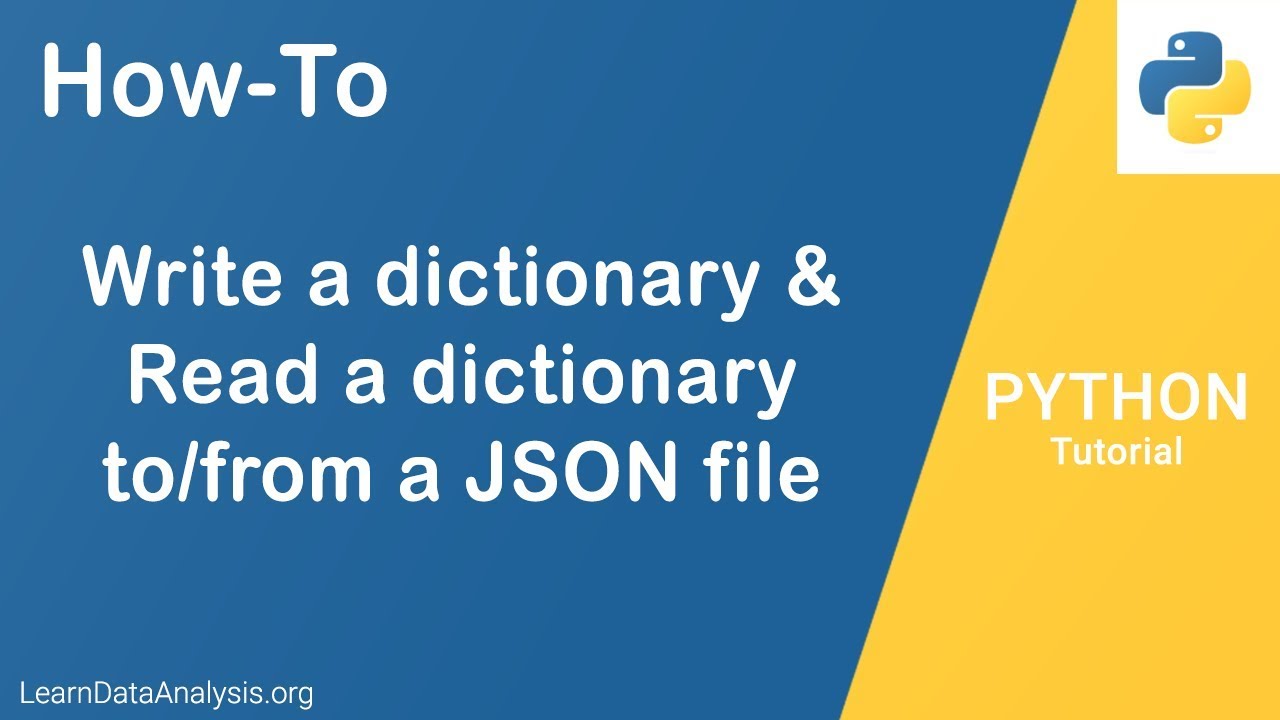
How do I save a dictionary to a file?
- dictionary_data = {“a”: 1, “b”: 2}
- a_file = open(“data.json”, “w”)
- json. dump(dictionary_data, a_file)
- a_file. close()
- a_file = open(“data.json”, “r”)
- output = a_file. read()
- print(output)
- a_file. close()
Can we write dictionary to a file in Python?
We can write dictionary to file by using json. dumps, for loop with dictionary items(), str(), keys() and pickle. dump() method.
Write and Read Dictionary To JSON file in Python | Python Tutorial
Images related to the topicWrite and Read Dictionary To JSON file in Python | Python Tutorial
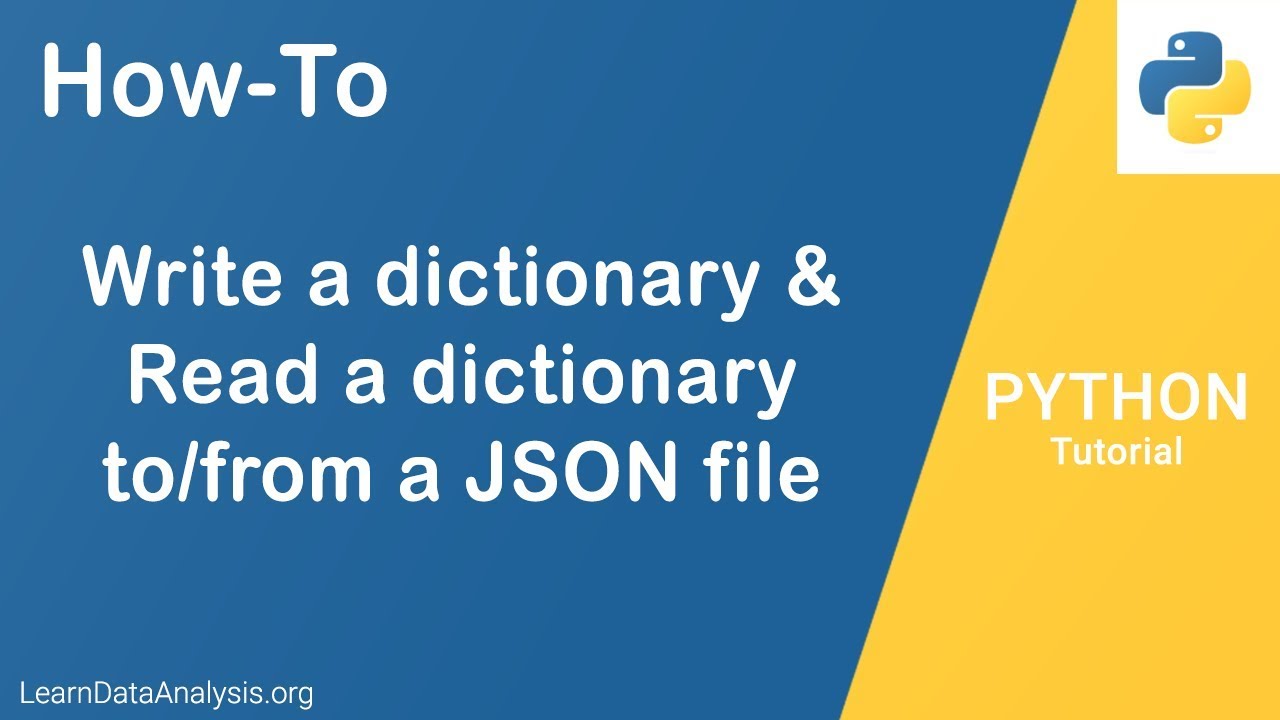
How do I print a dictionary file?
…
2. Pickle Module (Recommended)
- Importing the pickle module.
- Opening the file in write/append binary mode.
- Entering the data into the file using pickle module’s dump method.
How do I write a dictionary in CSV?
Easiest way is to open a csv file in ‘w’ mode with the help of open() function and write key value pair in comma separated form. The csv module contains DictWriter method that requires name of csv file to write and a list object containing field names.
Can you pickle a dictionary?
In general, pickling a dict will fail unless you have only simple objects in it, like strings and integers. Even a really simple dict will often fail.
How do I download a dictionary in Python?
- dict = {‘Python’ : ‘.py’, ‘C++’ : ‘.cpp’, ‘Java’ : ‘.java’}
- f = open(“dict.txt”,”w”)
- f. write( str(dict) )
- f. close()
How do you store a dictionary in Python?
In Python, a Dictionary can be created by placing a sequence of elements within curly {} braces, separated by ‘comma’. Dictionary holds pairs of values, one being the Key and the other corresponding pair element being its Key:value.
See some more details on the topic write dict to file here:
Save a dictionary to a file – GeeksforGeeks
Entering the converted string into the file using write function. filehandler = open(filename, ‘wt’) data = str(dictionary) filehander.write( …
Writing a dictionary to a text file? – Stack Overflow
First of all you are opening file in read mode and trying to write into it. Consult – IO modes python. Secondly, you can only write a string …
Write Dictionary To Text File In Python – DevEnum.com
The simple method to write a dictionary to a text file is by using the ‘for’ loop. First Open the file in write mode by using the File open() method. Then Get …
save dictionary python – Pythonspot
save dictionary as csv file. The csv module allows Python programs to write to and read from CSV (comma-separated value) files.
Can we convert dictionary to list in Python?
Convert Dictionary Into a List of Tuples in Python. A dictionary can be converted into a List of Tuples using two ways. One is the items() function, and the other is the zip() function.
How do you create a text file in Python?
- Step 1) Open the .txt file f= open(“guru99.txt”,”w+”) …
- Step 2) Enter data into the file for i in range(10): f.write(“This is line %d\r\n” % (i+1)) …
- Step 3) Close the file instance f.close() …
- Step 1) f=open(“guru99.txt”, “a+”)
How do I write a dictionary list to a text file in Python?
If your data will be only be accessed by python code, you can use shelve, here is an example: import shelve my_dict = {“foo”:”bar”} # file to be used shelf = shelve. open(“filename. shlf”) # serializing shelf[“my_dict”] = my_dict shelf.
How do I save a large dictionary in Python?
If you just want to work with a larger dictionary than memory can hold, the shelve module is a good quick-and-dirty solution. It acts like an in-memory dict, but stores itself on disk rather than in memory. shelve is based on cPickle, so be sure to set your protocol to anything other than 0.
How do you save a dictionary pickle?
Use pickle. dump() to save a dictionary to a file
Call open(file, mode) with the desired filename as file and “wb” as mode to open the Pickle file for writing in binary. Use pickle. dump(obj, file) with the dictionary as obj and the file object as file to store the dictionary in the file.
Simple Python Coder – File text into Python Dictionary
Images related to the topicSimple Python Coder – File text into Python Dictionary
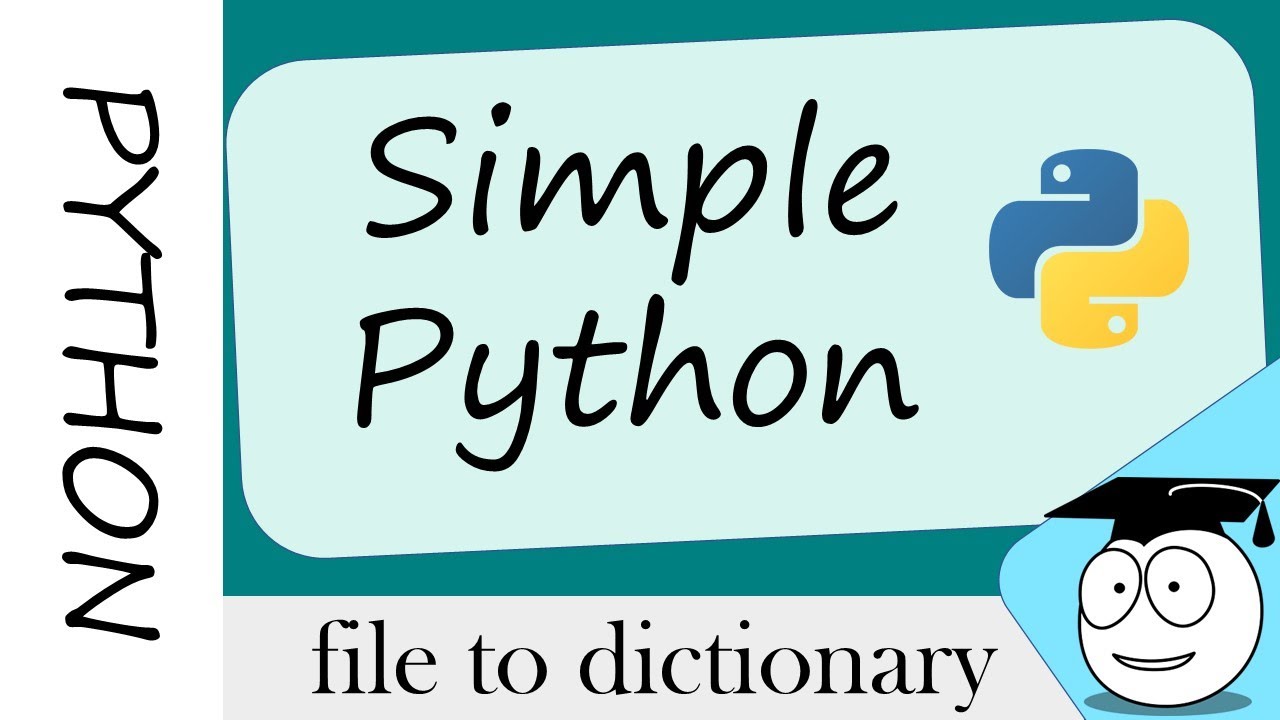
How do you turn a dictionary into a data frame?
We can convert a dictionary to a pandas dataframe by using the pd. DataFrame. from_dict() class-method.
How do I make a list into a csv file?
csv is the name of the file, the “w” mode is used to write the file, to write the list to the CSV file write = csv. writer(f), to write each row of the list to csv file writer. writerow() is used.
How do you create a CSV file?
- Open the workbook you want to save.
- Click File > Save As.
- Pick the place where you want to save the workbook.
- In the Save As dialog box, navigate to the location you want.
- Click the arrow in the Save as type box and pick the type of text or CSV file format you want.
Are pickles faster than JSON?
JSON is a lightweight format and is much faster than Pickling. There is always a security risk with Pickle. Unpickling data from unknown sources should be avoided as it may contain malicious or erroneous data. There are no loopholes in security using JSON, and it is free from security threats.
How do I create a pickle file?
- import pickle #credits to stack overflow user= blender.
- a = {‘hello’: ‘world’}
- with open(‘filename.pkl’, ‘wb’) as handle:
- pickle. dump(a, handle, protocol=pickle. HIGHEST_PROTOCOL)
- with open(‘filename.pkl’, ‘rb’) as handle:
- b = pickle. load(handle)
What is pickle file?
Pickle can be used to serialize Python object structures, which refers to the process of converting an object in the memory to a byte stream that can be stored as a binary file on disk. When we load it back to a Python program, this binary file can be de-serialized back to a Python object.
How do you save a dict object in Python?
- # define dict.
- dict = {‘Python’ : ‘.py’, ‘C++’ : ‘.cpp’, ‘Java’ : ‘.java’}
- # open file for writing.
- # write file.
- # close file.
How do I import an Oxford dictionary into Python?
- Download a JSON file containing English dictionary words in a python dictionaries data type format, or arrange the file content in that way.
- Create a folder and add the downloaded . json file and python script in that folder.
- In python editor, import the required modules.
How do you pickle a dictionary in Python?
- import pickle. # save dictionary to pickle file. with open(‘my_filename.pickle’, ‘wb’) as file: pickle. …
- import pickle. # create a dictionary. employees = { “Jim”: “Sales”, …
- # laod a pickle file. with open(“employee_info.pickle”, “rb”) as file: loaded_dict = pickle. load(file)
How do you print a dictionary key in Python?
To print the dictionary keys in Python, use the dict. keys() method to get the keys and then use the print() function to print those keys. The dict. keys() method returns a view object that displays a list of all the keys in the dictionary.
022g Writing a list of dictionaries to a CSV file
Images related to the topic022g Writing a list of dictionaries to a CSV file
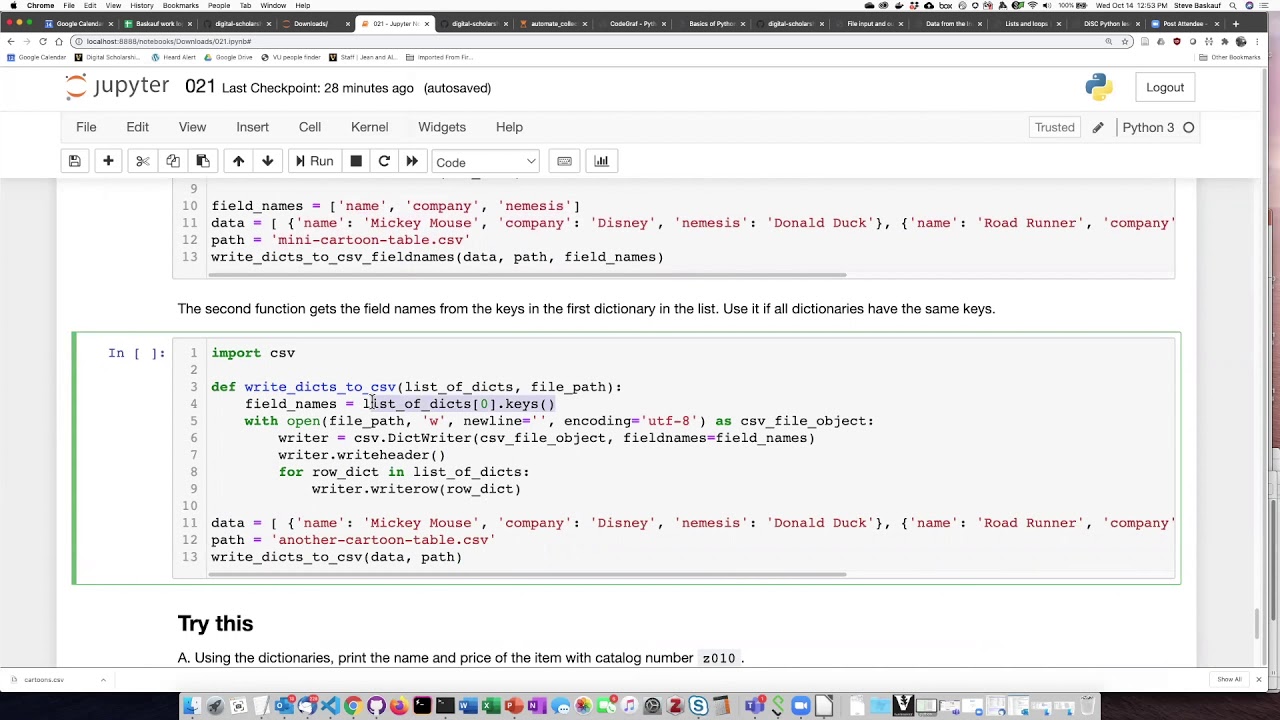
How do you print a dictionary value in Python?
Print a dictionary line by line using for loop & dict. items() dict. items() returns an iterable view object of the dictionary that we can use to iterate over the contents of the dictionary, i.e. key-value pairs in the dictionary and print them line by line i.e.
How do you create a dictionary in a list?
To convert a list to a dictionary using the same values, you can use the dict. fromkeys() method. To convert two lists into one dictionary, you can use the Python zip() function. The dictionary comprehension lets you create a new dictionary based on the values of a list.
Related searches to write dict to file
- write dict to file python
- python read write dict to file
- txt to dictionary python
- write list to file python
- write json dict to file python
- append dict to file python
- Write dict to file Python
- ansible write dict to file
- python write dict to file as csv
- Write list to file python
- python write dict to file pretty
- write dict to file python pickle
- python write dict to file with double quotes
- pandas write dict to file
- tcl write dict to file
- Save dict to file python
- save dict to file python
- python write dict to file
- yaml write dict to file
- Pickle dump to file
- write dict to file as json python
- append value to key in dictionary python
- Append value to key in dictionary Python
- Append dict to file Python
- write list to file txt
- python write dict to file as json
- pickle dump to file
- write list of dict to file python
Information related to the topic write dict to file
Here are the search results of the thread write dict to file from Bing. You can read more if you want.
You have just come across an article on the topic write dict to file. If you found this article useful, please share it. Thank you very much.