Are you looking for an answer to the topic “typescript casting“? We answer all your questions at the website Chambazone.com in category: Blog sharing the story of making money online. You will find the answer right below.
There are times when working with types where it’s necessary to override the type of a variable, such as when incorrect types are provided by a library. Casting is the process of overriding a type.Typescript is about typings, but you always have a way to break the typing for good or bad reasons. So here are some key advices. Always type (not cast) all your code but forget the any as much as possible. If you have to cast, it’s a polymorphism-like reason, or it’s probably a code smell.In programming language, casting is a way of telling the compiler to change an expression or value from one type to another. At times, you may want to convert your JavaScript expressions or values from one type to another.
- const person = { firstName: ‘John’, lastName: ‘Doe’ }; …
- const propertyNames = Object.keys(person); console.log(propertyNames); …
- const propertyValues = Object.values(person); console.log(propertyValues); …
- const entries = Object.entries(person); console.log(entries);
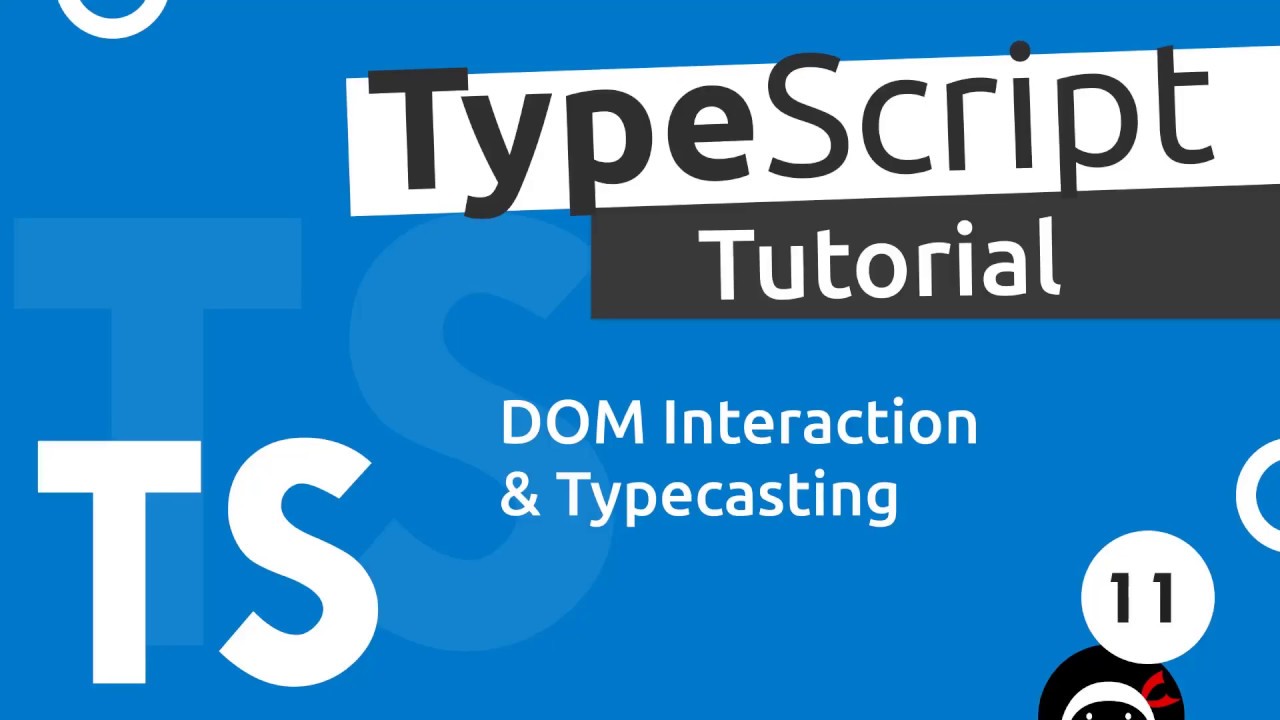
Is type casting bad in TypeScript?
Typescript is about typings, but you always have a way to break the typing for good or bad reasons. So here are some key advices. Always type (not cast) all your code but forget the any as much as possible. If you have to cast, it’s a polymorphism-like reason, or it’s probably a code smell.
What is casting in JavaScript?
In programming language, casting is a way of telling the compiler to change an expression or value from one type to another. At times, you may want to convert your JavaScript expressions or values from one type to another.
TypeScript Tutorial #11 – The DOM Type Casting
Images related to the topicTypeScript Tutorial #11 – The DOM Type Casting
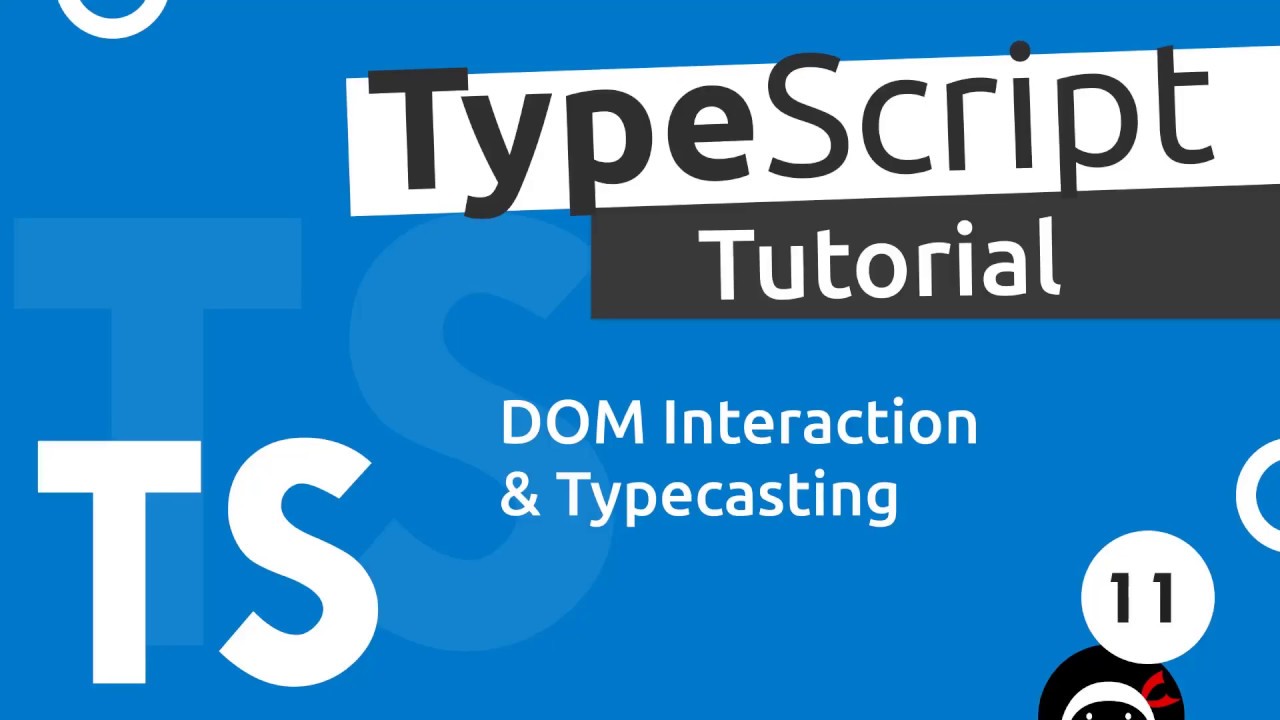
What is datatype casting?
Type casting refers to changing an variable of one data type into another. The compiler will automatically change one type of data into another if it makes sense. For instance, if you assign an integer value to a floating-point variable, the compiler will convert the int to a float.
How do you cast an object in JavaScript?
- const person = { firstName: ‘John’, lastName: ‘Doe’ }; …
- const propertyNames = Object.keys(person); console.log(propertyNames); …
- const propertyValues = Object.values(person); console.log(propertyValues); …
- const entries = Object.entries(person); console.log(entries);
Is casting code smell?
Explicit casts. I wish there was a compiler option that could make it flag these as warnings (or even better, errors). These hateful things sit there like timebombs in your code, just waiting for an opportunity to explode in a shower of ClassCastExceptions.
How do I cast objects in TypeScript?
Then you can use it to force cast objects to a certain type: import { forceCast } from ‘./forceCast’; const randomObject: any = {}; const typedObject = forceCast<IToDoDto>(randomObject);
How do you cast a string in JavaScript?
The toString() method in Javascript is used with a number and converts the number to a string. It is used to return a string representing the specified Number object. The toString() method is used with a number num as shown in above syntax using the ‘. ‘ operator.
See some more details on the topic typescript casting here:
Type Casting – TypeScript Tutorial
Type casting allows you to convert a variable from one type to another. Use the as keyword or <> operator for type castings. Was this tutorial helpful ?
TypeScript or JavaScript type casting – Stack Overflow
You can cast like this: return this.createMarkerStyle(
Typescript cast is a type breaker – DEV Community
Typescript offers the “cast” feature to convert from a type to another. But using it wrong can break the type-check of your code.
Type casting in Typescript – Techformist
The process of changing types is what is called “type casting”. Typecasting in strongly typed languages like C# allow us to convert types.
How do I cast a number in JavaScript?
- Using parseInt() parseInt() parses a string and returns a whole number. …
- Using Number() Number() can be used to convert JavaScript variables to numbers. …
- Using Unary Operator (+) …
- Using parseFloat() …
- Using Math. …
- Multiply with number. …
- Double tilde (~~) Operator.
How many types of JavaScript are there?
In Javascript, there are five basic, or primitive, types of data. The five most basic types of data are strings, numbers, booleans, undefined, and null. We refer to these as primitive data types.
Is type casting bad?
Overall, being “type-cast” is not necessarily a bad thing, in fact it helps Hollywood produce quality movies. Actors and actresses hone a craft and make a certain type of character and role theirs and it improves the movies they make.
What is casting in programming?
Casting means changing the data type of a piece of data from one type to another. The data may be stored inside a variable. To ask the user to input a number, you might use this code: number = INPUT(“Please enter a number”)
What is difference between type casting and type conversion?
1. In type casting, a data type is converted into another data type by a programmer using casting operator. Whereas in type conversion, a data type is converted into another data type by a compiler.
TypeScript: Type Assertion or Type Casting
Images related to the topicTypeScript: Type Assertion or Type Casting

How do I cast an array in TypeScript?
There are 4 possible conversion methods in TypeScript for arrays: let x = []; //any[] let y1 = x as number[]; let z1 = x as Array<number>; let y2 = <number[]>x; let z2 = <Array<number>>x; The as operator’s mostly designed for *.
How do I iterate over an object in JavaScript?
- const items = { ‘first’: new Date(), ‘second’: 2, ‘third’: ‘test’ }
- items. map(item => {})
- items. forEach(item => {})
- for (const item of items) {}
- for (const item in items) { console. log(item) }
- Object. entries(items). map(item => { console. log(item) }) Object.
How do you implement type casting in Java?
- Widening Casting (automatically) – converting a smaller type to a larger type size. byte -> short -> char -> int -> long -> float -> double.
- Narrowing Casting (manually) – converting a larger type to a smaller size type. double -> float -> long -> int -> char -> short -> byte.
Is casting inefficient?
No, casts are fast – as little as a single instruction. The cast itself is not your problem. micros() returns an unsigned long. Any math done on the return value with less than an unsigned long is highly likely to give an incorrect result due to truncation and/or wrap-around.
What is code smell in Java?
Martin Fowler in his book Refactoring: Improving the design of existing code defines a code smell as: A surface indication that usually corresponds to a deeper problem in the system. Refactoring is a process of improving the internal structure of our code without impacting its external behavior.
Which does not require type casting?
char c; int i = 65; c = i; There is no need for a cast in this assignment because the integer type int of variable i is implicitly converted to the integer type of the destination.
What is difference between interface and type in TypeScript?
The typescript type supports only the data types and not the use of an object. The typescript interface supports the use of the object. Type keyword when used for declaring two different types where the variable names declared are the same then the typescript compiler will throw an error.
How do I Stringify a JSON object in TypeScript?
- Use the JSON.stringify() Method to Convert an Object Into a JSON String in TypeScript.
- Use JSON.stringify() and JSON.parse() to Convert an Object Into a JSON String in TypeScript.
How do I convert a string to a number in TypeScript?
In typescript, there are numerous ways to convert a string to a number. We can use the ‘+’ unary operator , Number(), parseInt() or parseFloat() function to convert string to number.
How do you cast a value to a string?
…
The three approaches for converting to string are:
- value. toString()
- “” + value.
- String(value)
43. DOM Typecasting in the Typescript. Cast Values in the Typescript.
Images related to the topic43. DOM Typecasting in the Typescript. Cast Values in the Typescript.
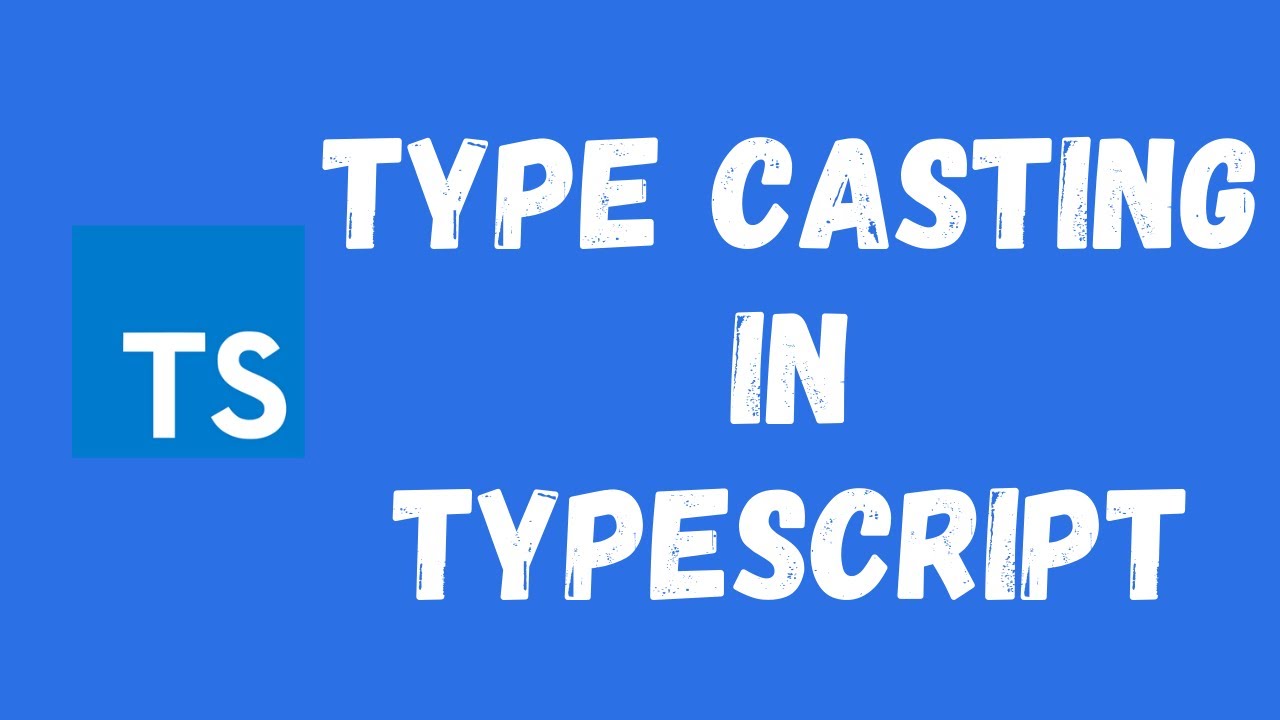
How do you cast a variable to a string?
Converting Numbers to Strings
We can convert numbers to strings through using the str() method. We’ll pass either a number or a variable into the parentheses of the method and then that numeric value will be converted into a string value.
What is toString in JavaScript?
toString . For user-defined Function objects, the toString method returns a string containing the source text segment which was used to define the function. JavaScript calls the toString method automatically when a Function is to be represented as a text value, e.g. when a function is concatenated with a string.
Related searches to typescript casting
- typescript casting not working
- typescript casting to string
- typescript type casting
- Convert object to type typescript
- angular typescript casting
- typescript conditional casting
- convert any to array typescript
- typescript casting string to number
- typescript casting vs as
- eslint typescript casting
- typescript casting array
- typescript casting html element
- typescript casting types
- typescript casting react
- typescript dynamic type casting
- tuple in typescript
- typescript force casting
- Convert any to array typescript
- typescript casting object
- never typescript
- typescript safe type casting
- type in typescript
- typescript type casting array
- react typescript casting
- For-in object TypeScript
- Tuple in TypeScript
- typescript type casting not working
- convert object to type typescript
- convert number to string typescript
- how to convert any to object in typescript
- typescript type casting object
- Type in TypeScript
- for in object typescript
- typescript interface casting
- Convert number to string typescript
- typescript inline type casting
Information related to the topic typescript casting
Here are the search results of the thread typescript casting from Bing. You can read more if you want.
You have just come across an article on the topic typescript casting. If you found this article useful, please share it. Thank you very much.