Are you looking for an answer to the topic “typescript check array type“? We answer all your questions at the website Chambazone.com in category: Blog sharing the story of making money online. You will find the answer right below.
Keep Reading
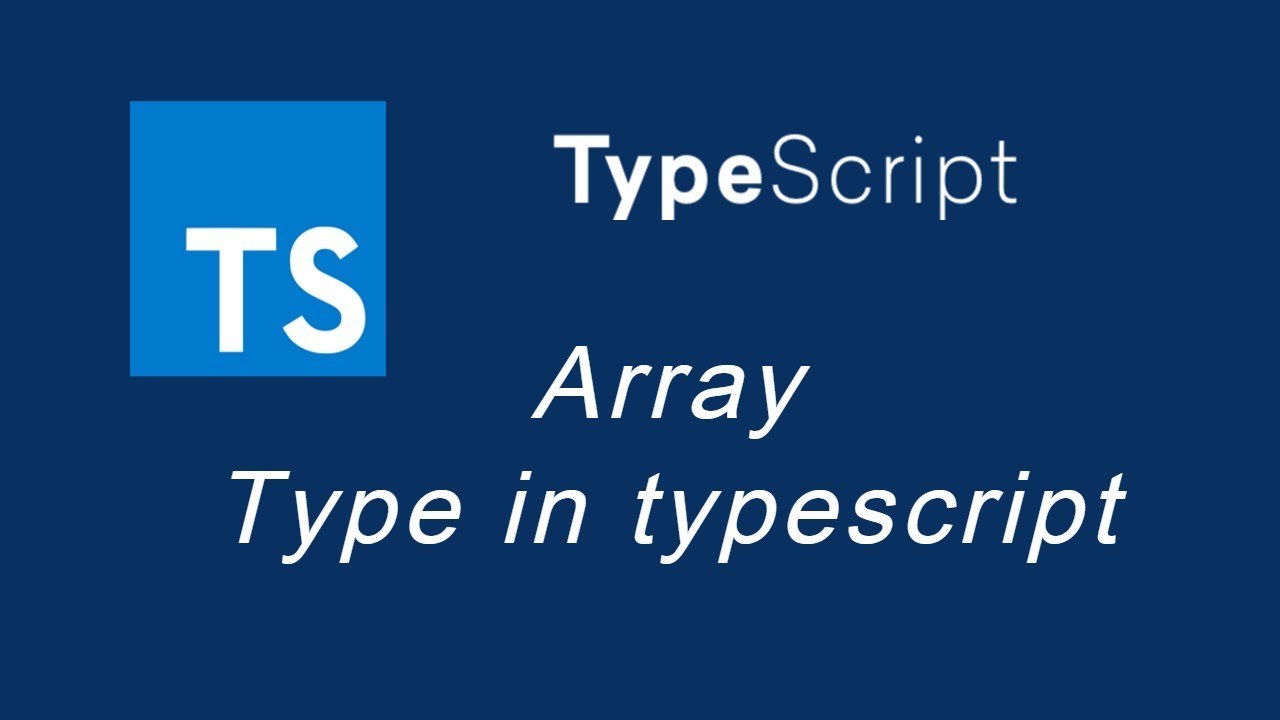
How do I know the type of an array?
Answer: Use the Array. isArray() Method
isArray() method to check whether an object (or a variable) is an array or not. This method returns true if the value is an array; otherwise returns false .
What is the type for array in TypeScript?
An array in TypeScript can contain elements of different data types using a generic array type syntax, as shown below. let values: (string | number)[] = [‘Apple’, 2, ‘Orange’, 3, 4, ‘Banana’]; // or let values: Array<string | number> = [‘Apple’, 2, ‘Orange’, 3, 4, ‘Banana’];
Typescript tutorial #9 Array Type in typescript
Images related to the topicTypescript tutorial #9 Array Type in typescript
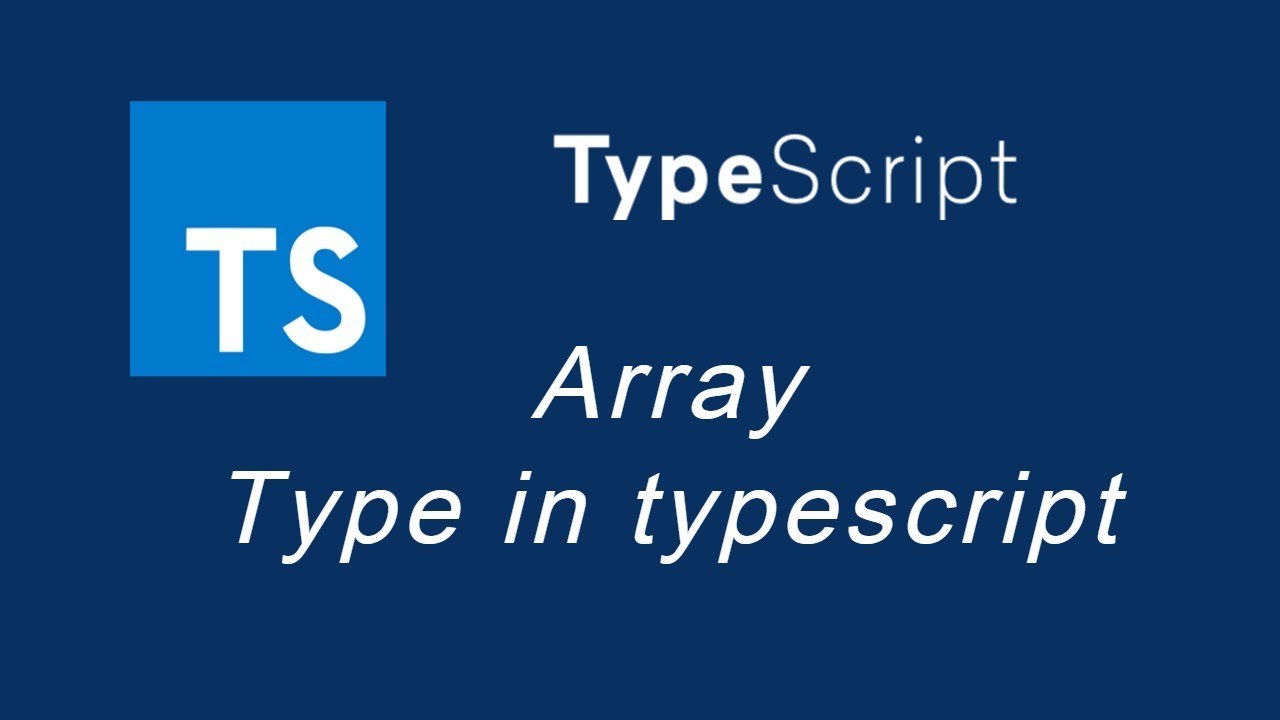
How do you check if a variable is a list in TypeScript?
- function isString(value) {
- return typeof value === ‘string’ || value instanceof String;
- }
-
- isString(”); // true.
- isString(1); // false.
- isString({}); // false.
- isString([]); // false.
How do you check if a variable is an array of strings?
The Array. isArray() method checks whether the passed variable is an Array object. It returns a true boolean value if the variable is an array and false if it is not. This is shown in the example below.
What is the type of array in JavaScript?
Js array is divided into two types: One-dimensional arrays. Multi-dimensional arrays.
What is Array type of array?
An array type is a user-defined data type consisting of an ordered set of elements of a single data type. An ordinary array type has a defined upper bound on the number of elements and uses the ordinal position as the array index.
Where are TypeScript types defined?
TypeScript automatically finds type definitions under node_modules/@types , so there’s no other step needed to get these types available in your program.
See some more details on the topic typescript check array type here:
Check if a Value is an Array (of type) in TypeScript | bobbyhadz
To check if a value is an array of specific type in TypeScript, use the `Array.isArray()` method to check if the value is an array.
TypeScript: How to get types from arrays | Steve Holgado
How to get types from arrays with TypeScript. … It can be useful to get a type from an array of values so that you can use it as, say, the input to a …
Array.isArray() – JavaScript – MDN Web Docs
The Array.isArray() method determines whether the passed value is an Array.
typescript check if array Code Example – Grepper
“typescript check if array” Code Answer’s ; javascript check if is array. javascript by Grepper on Jul 22 2019 Donate Comment. 13 · 1 ; javascript typeof array.
How do you test if a variable is an array in JavaScript?
isArray(variableName) method to check if a variable is an array. The Array. isArray(variableName) returns true if the variableName is an array. Otherwise, it returns false .
What are TypeScript types?
Built-in Data Type | keyword | Description |
---|---|---|
Number | number | It is used to represent both Integer as well as Floating-Point numbers |
Boolean | boolean | Represents true and false |
String | string | It is used to represent a sequence of characters |
Void | void | Generally used on function return-types |
How do you check if an object is an array or not?
isArray() method is used to check if an object is an array. The Array. isArray() method returns true if an object is an array, otherwise returns false .
How do you check if an object is present in an array in TypeScript?
- var arr = [{ id: 1, name: ‘JOHN’ },
- { id: 2, name: ‘JENNIE’},
- { id: 3, name: ‘JENNAH’ }];
-
- function userExists(name) {
- return arr. some(function(el) {
- return el. name === name;
- });
Is array a data type in JavaScript?
yes , Array is not a data type in JavaScript. The set of types in JavaScript are of Primitive values and Objects. So Array falls under the category of Objects.
TypeScript Tutorial #4 – Objects Arrays
Images related to the topicTypeScript Tutorial #4 – Objects Arrays
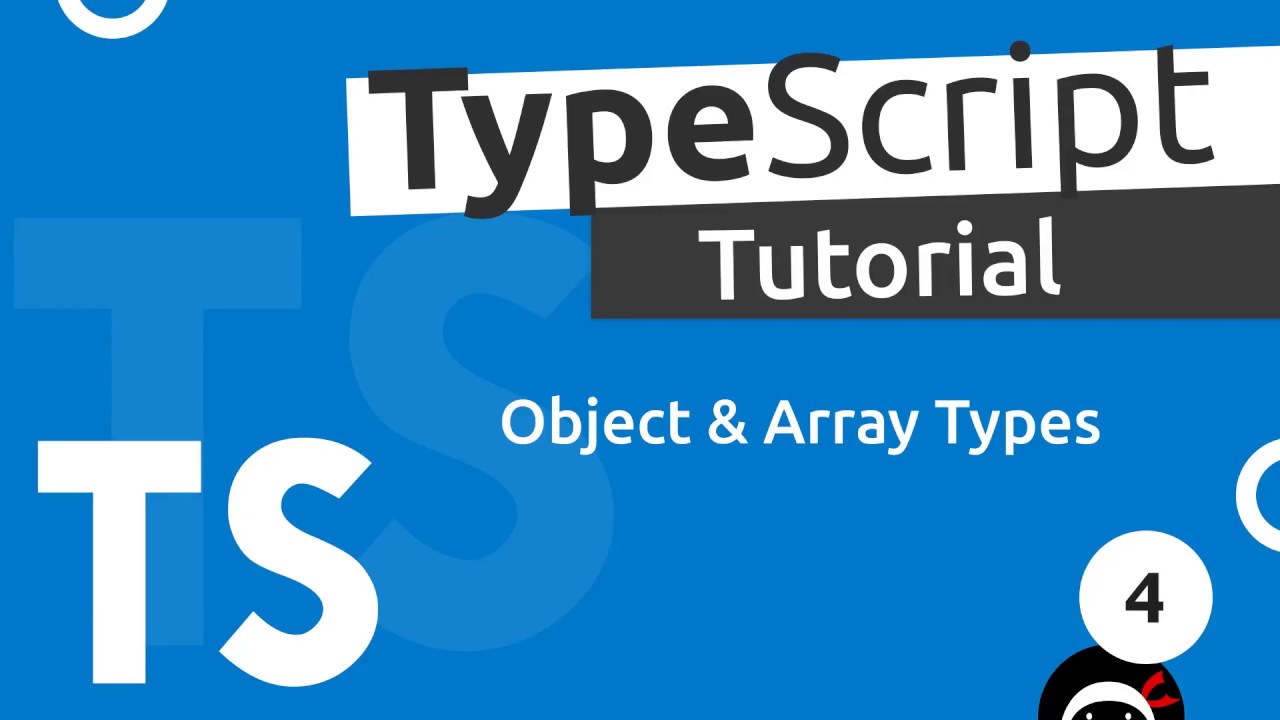
How do you use typeof?
Real-world usage
typeof is very useful, but it’s not as versatile as might be required. For example, typeof([]) , is ‘object’ , as well as typeof(new Date()) , typeof(/abc/) , etc. For checking non-existent variables that would otherwise throw a ReferenceError , use typeof nonExistentVar === ‘undefined’ .
How do you check if a value is not in an array JavaScript?
The indexof() method in Javascript is one of the most convenient ways to find out whether a value exists in an array or not. The indexof() method works on the phenomenon of index numbers. This method returns the index of the array if found and returns -1 otherwise.
Is array a polyfill?
The most important reason that makes the MDN polyfill a safe one to use is that an array is an exotic object. The best way to differentiate an exotic object would be through the use of an exotic feature related to that object – that is to say, an exotic property of the exotic object.
Why typeof array is object in JavaScript?
Apparently there seems to be something wrong because the array is recognized as an object and seems to be no real difference between object and array. This because in javascript all derived data type is always a type object. Included functions and array.
How do you create a typed array?
- // create a TypedArray with a size in bytes.
- const typedArray1 = new Int8Array(8);
- typedArray1[0] = 32;
- const typedArray2 = new Int8Array(typedArray1);
- typedArray2[1] = 42;
- console. log(typedArray1);
How do I use TypedArray?
To use Typed Arrays, you need to create an ArrayBuffer and a view to it. The easiest way is to create a typed array view of the desired size and type. // Typed array views work pretty much like normal arrays. var f64a = new Float64Array(8); f64a[0] = 10; f64a[1] = 20; f64a[2] = f64a[0] + f64a[1];
How do you access an array element?
Array elements are accessed by using an integer index. Array index starts with 0 and goes till size of array minus 1. Name of the array is also a pointer to the first element of array.
What are the types of data types?
- Integer (int) It is the most common numeric data type used to store numbers without a fractional component (-707, 0, 707).
- Floating Point (float) …
- Character (char) …
- String (str or text) …
- Boolean (bool) …
- Enumerated type (enum) …
- Array. …
- Date.
What is 1d array?
Definition. A One-Dimensional Array is the simplest form of an Array in which the elements are stored linearly and can be accessed individually by specifying the index value of each element stored in the array.
Where are TypeScript types stored?
- Ambient typing. Ie. Global *. …
- You can store interfaces directly on the main file that use them.
- Like any other class, function or object, you can explicitly export and import types from . ts files.
TypeScript Part9- Arrays in TypeScript
Images related to the topicTypeScript Part9- Arrays in TypeScript

What does => mean in TypeScript?
In a type position, => defines a function type where the arguments are to the left of the => and the return type is on the right.
What is ?: operator in TypeScript?
TypeScript 3.7 added support for the ?? operator, which is known as the nullish coalescing operator. We can use this operator to provide a fallback value for a value that might be null or undefined .
Related searches to typescript check array type
- typescript check type and cast
- check if variable is array javascript
- typescript any of array
- typescript types list
- typescript check if variable is type array
- typescript check if generic type is array
- typescript check type of array element
- typescript instanceof array
- typescript types number
- type of array javascript
- typescript check if array contains type
- typescript type array of objects
- typescript check if type extends array
- typescript check if list
- typescript array.isarray not working
- typescript all types
- typescript array pop all
- typescript check if typeof array
- typescript list of
- typescript array isarray not working
- typescript check type
- typescript check if parameter is of type array
Information related to the topic typescript check array type
Here are the search results of the thread typescript check array type from Bing. You can read more if you want.
You have just come across an article on the topic typescript check array type. If you found this article useful, please share it. Thank you very much.