Are you looking for an answer to the topic “typescript hasownproperty“? We answer all your questions at the website Chambazone.com in category: Blog sharing the story of making money online. You will find the answer right below.
Keep Reading
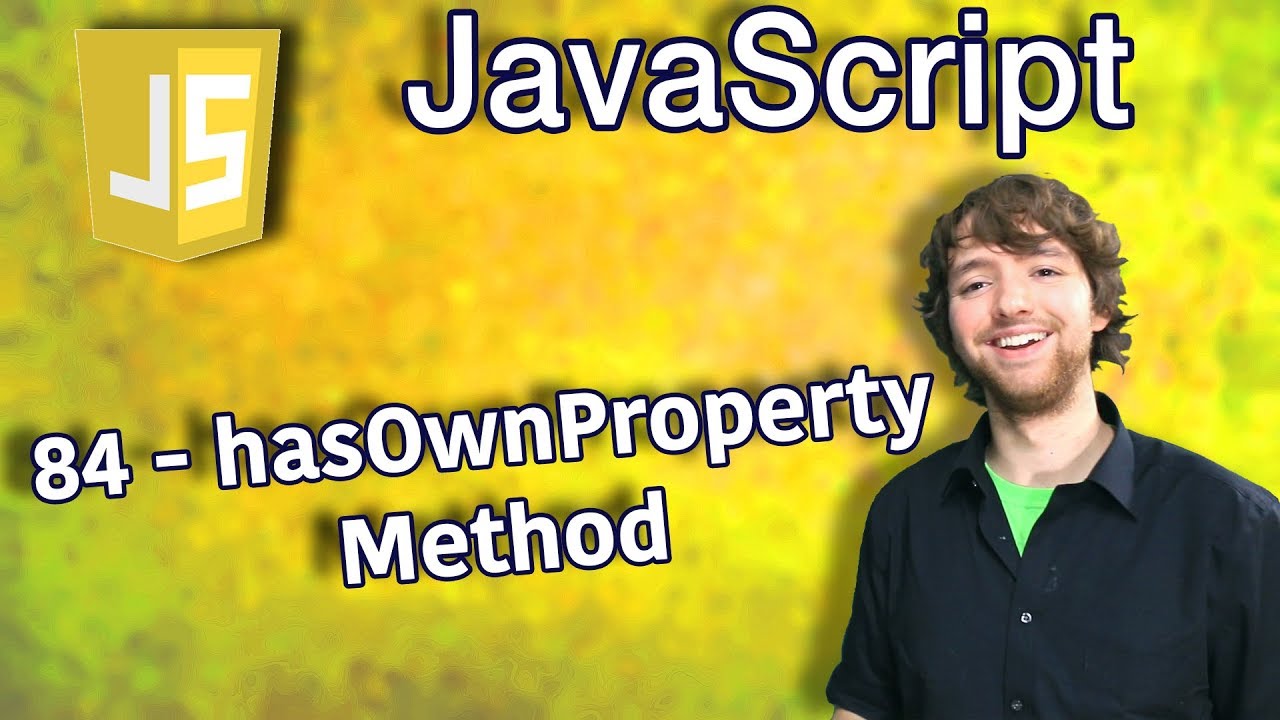
What is hasOwnProperty TypeScript?
hasOwnProperty() The hasOwnProperty() method returns a boolean indicating whether the object has the specified property as its own property (as opposed to inheriting it).
How do I use hasOwnProperty in TypeScript?
- var person = {
- “first_name”: “Bob”,
- “last_name”: “Dylan”
- };
- person. hasOwnProperty(‘first_name’); //returns true.
- person. hasOwnProperty(‘age’); //returns false.
JavaScript Programming Tutorial 84 – hasOwnProperty Method
Images related to the topicJavaScript Programming Tutorial 84 – hasOwnProperty Method
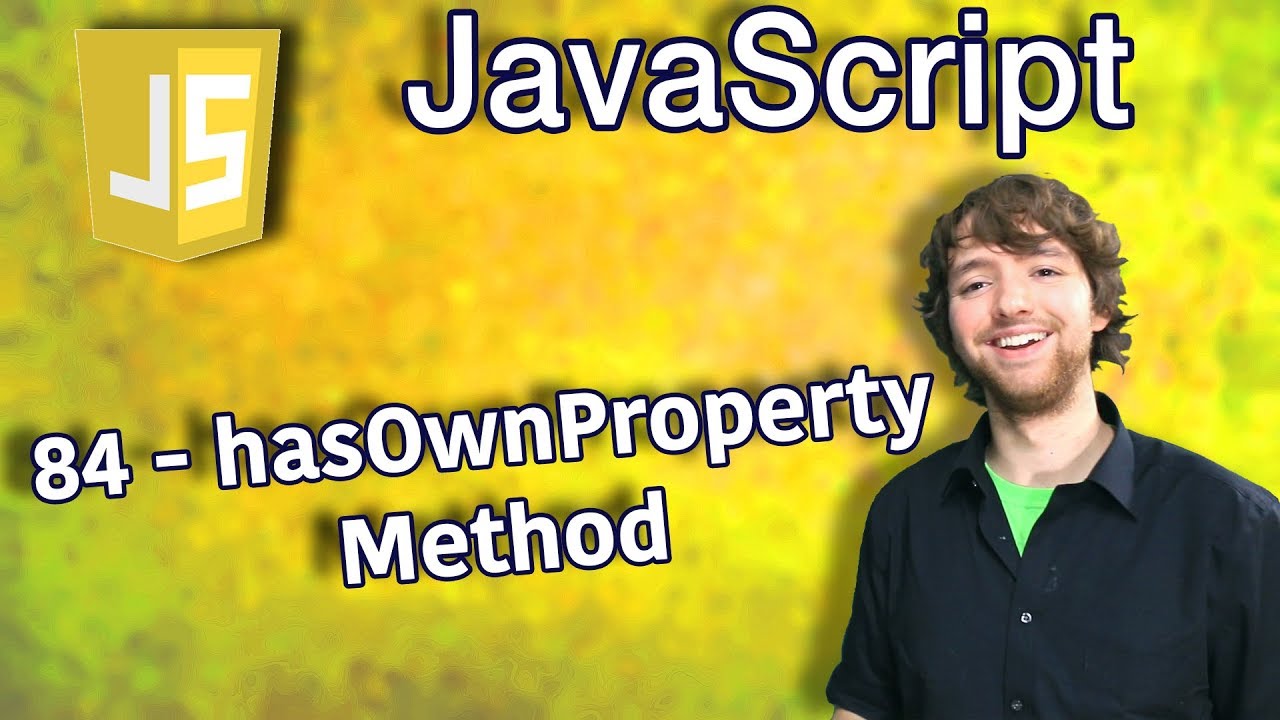
Should I use hasOwnProperty?
A good rule of thumb is that if you’re looking to see whether an object has a property, you should use hasOwnProperty() . If you’re looking to see if an object has a function that you intend to call, like checking if an object has toString() , you should use in .
How do you check if an object has a property in TypeScript?
The first way is to invoke object. hasOwnProperty(propName) . The method returns true if the propName exists inside object , and false otherwise.
How do you check if an object is empty?
- Pass the object to the Object. keys method to get an array of all the keys of the object.
- Access the length property on the array.
- Check if the length of keys is equal to 0 , if it is, then the object is empty.
How do you check if an object is empty in TypeScript?
- Use the Object. keys() method to get an array of the object’s keys.
- Access the length property on the array.
- If the length property is equal to 0 , the object is empty.
How do you check if an object includes a value?
Use the Object.
values method to return an array of property values of an object. Therefore, we can use that to check if a value exists in an object. We call indexOf on the array of property values that are returned by Object.
See some more details on the topic typescript hasownproperty here:
Object.prototype.hasOwnProperty() – JavaScript – MDN Web …
The hasOwnProperty() method returns true if the specified property is a direct property of the object — even if the value is null or …
TypeScript hasOwnProperty equivalent – javascript – Stack …
If obj is a TypeScript object (instance of a class), is there a way to perform the same operation? Your JavaScript is valid TypeScript …
TypeScript: Check for object properties and narrow down type
TypeScript’s control flow analysis lets you narrow down from a broader type … function hasOwnProperty
The Uses of ‘in’ vs ‘hasOwnProperty’ – A Drip of JavaScript
The in operator will tell you whether an object (or array) has a property name which matches a given string. var fantasyLit = { …
How do you iterate over an object in JavaScript?
- const items = { ‘first’: new Date(), ‘second’: 2, ‘third’: ‘test’ }
- items. map(item => {})
- items. forEach(item => {})
- for (const item of items) {}
- for (const item in items) { console. log(item) }
- Object. entries(items). map(item => { console. log(item) }) Object.
How do you add a property to an object?
- var obj = { Name: “Joe” };
- obj. Age = 12;
- console. log(obj. Age)
- obj[‘Country’] = “USA”
- console. log(obj. Country)
What is the difference between in and hasOwnProperty?
in vs hasOwnProperty
The key difference is that in will return true for inherited properties, whereas hasOwnProperty() will return false for inherited properties. For example, the base Object in JavaScript has a toString property, a constructor property and a couple others.
Are object keys fast?
Also, Object. keys is a native function. Hence, in all probability it is faster than a JavaScript shim for the same.
Is property of object JavaScript?
JavaScript is designed on a simple object-based paradigm. An object is a collection of properties, and a property is an association between a name (or key) and a value. A property’s value can be a function, in which case the property is known as a method.
Check if a property is in an object – Beau teaches JavaScript
Images related to the topicCheck if a property is in an object – Beau teaches JavaScript
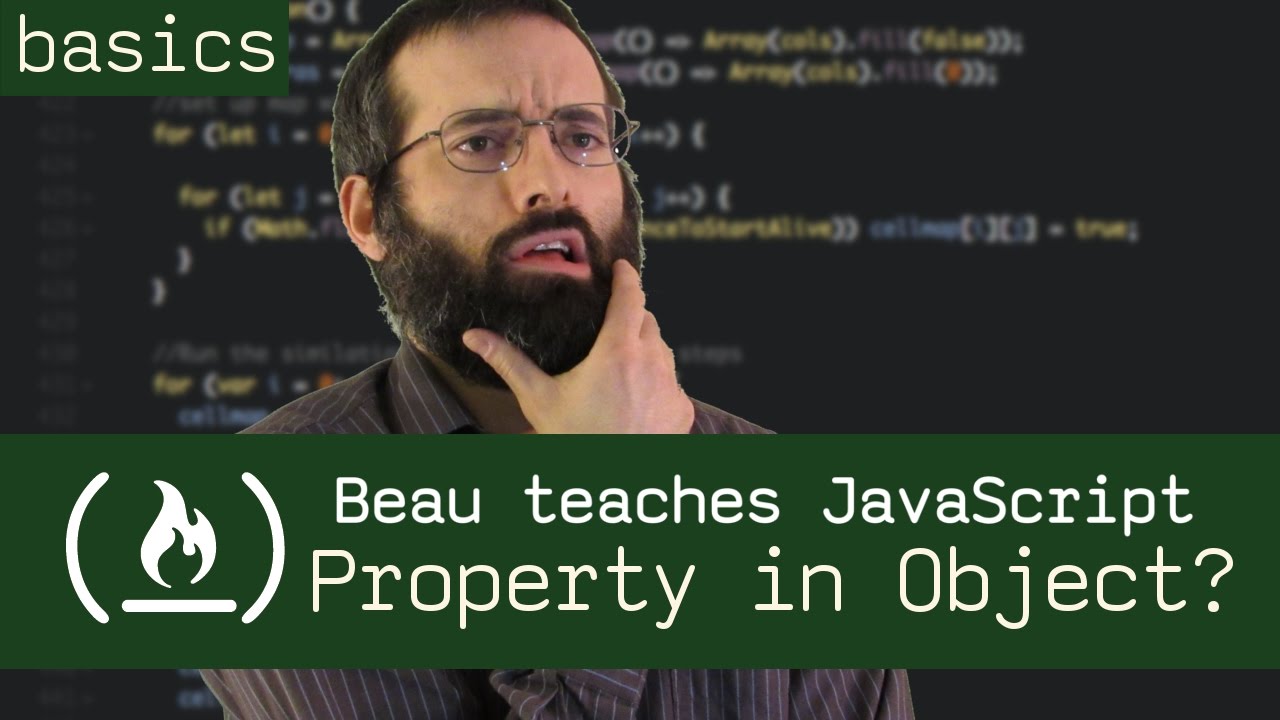
How do you know if an object has moved?
Motion Physics. An object is in motion when its distance from another object is changing. Whether an object is moving or not depends on your point of view. For example, a woman riding on a bus is not moving in relation to the seat she is sitting on, but she is moving in relation to the buildings the bus passes.
How do you know if an object has changed position?
changes position requires a point of reference. An object changes position if it moves relative to a reference point. To visualize this, picture yourself competing in a 100-m dash.
What is property in JavaScript?
A JavaScript property is a characteristic of an object, often describing attributes associated with a data structure. There are two kinds of properties: Instance properties hold data that are specific to a given object instance. Static properties hold data that are shared among all object instances.
How do I create an empty object in typescript?
- type User = {
- Username: string;
- Email: string;
- }
-
- const user01 = {} as User;
- const user02 = <User>{};
-
Is an empty object truthy?
Values not on the list of falsy values in JavaScript are called truthy values and include the empty array [] or the empty object {} . This means almost everything evaluates to true in JavaScript — any object and almost all primitive values, everything but the falsy values.
How do you check is JSON empty or not?
return Object.keys(obj).length === 0 ;
This is typically the easiest way to determine if an object is empty.
How check string is empty in typescript?
Use the === Operator to Check if the String Is Empty in JavaScript. We can use the strict equality operator ( === ) to check whether a string is empty or not. The comparison data===”” will only return true if the data type of the value is a string, and it is also empty; otherwise, return false .
How can you tell if an object is undefined?
In a JavaScript program, the correct way to check if an object property is undefined is to use the `typeof` operator. See how you can use it with this simple explanation. In a JavaScript program, the correct way to check if an object property is undefined is to use the typeof operator.
How do I check if an array is empty in typescript?
- const users: Array<Object> = [];
- if(typeof users !== “undefined” && users. length == 0){
- console. log(“Array is empty”);
How do I check if an object contains a string?
The includes() method returns true if a string contains a specified string. Otherwise it returns false .
Typescript: 01-04 – Setup unit test với ts-jest 🔥
Images related to the topicTypescript: 01-04 – Setup unit test với ts-jest 🔥
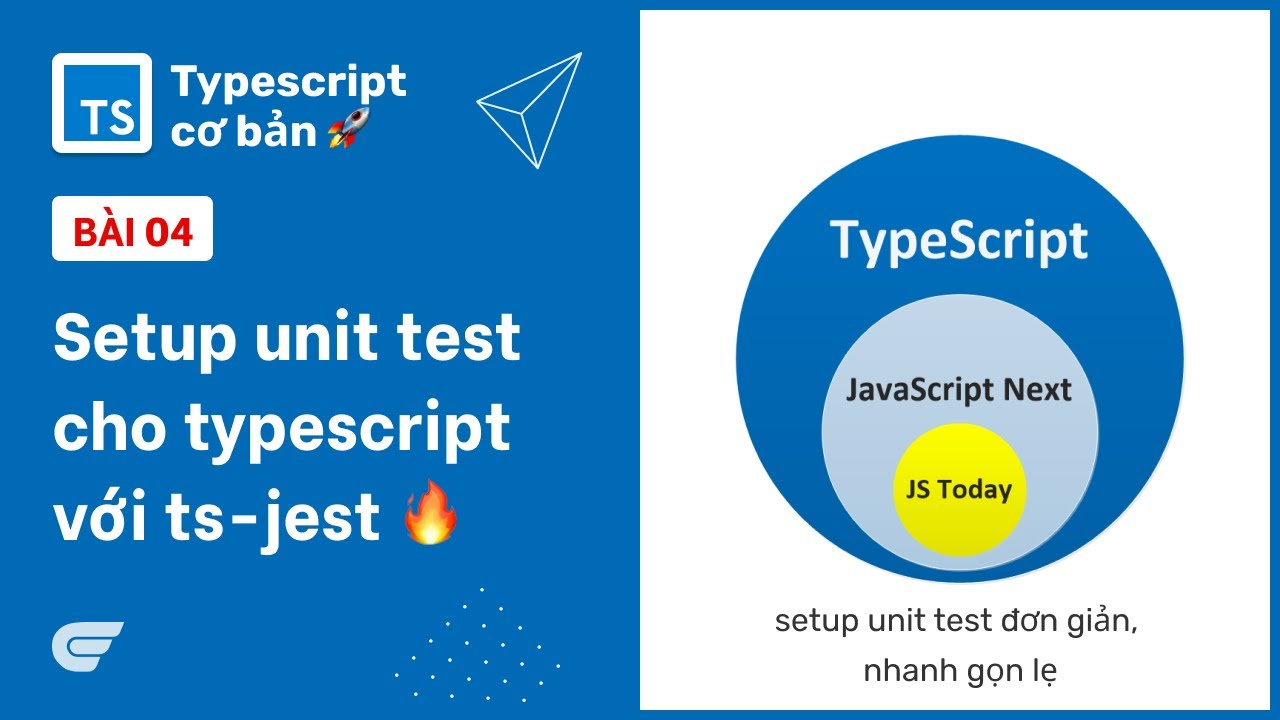
How do you check if an object includes a value in JavaScript?
Check for object value using Object. values()
We can check if a value exists in an object using Object. values() . We can use includes() to check for the value. Or, we can use indexOf() .
How do you check if a value exists in an object using JavaScript?
You can use Object. values(): and then use the indexOf() method: Show activity on this post.
Related searches to typescript hasownproperty
- typescript any hasownproperty
- typescript unknown hasownproperty
- typescript json hasownproperty
- typescript hasownproperty check
- do not access object prototype method hasownproperty from target object
- typescript check if object has property
- eslint hasownproperty
- typescript hasownproperty does not exist
- typescript generic hasownproperty
- typescript hasownproperty getter
- javascript hasownproperty
- typescript hasownproperty alternative
- typescript hasownproperty vs in
- typescript interface hasownproperty
- typescript enum hasownproperty
- typescript object.prototype.hasownproperty.call
- typescript hasownproperty get value
- typescript hasownproperty type guard
- typescript lint hasownproperty
- angular typescript hasownproperty
- typescript object hasownproperty
- hasownproperty w3schools
- typescript in vs hasownproperty
- hasownproperty is not a function
Information related to the topic typescript hasownproperty
Here are the search results of the thread typescript hasownproperty from Bing. You can read more if you want.
You have just come across an article on the topic typescript hasownproperty. If you found this article useful, please share it. Thank you very much.