Are you looking for an answer to the topic “typescript key value list“? We answer all your questions at the website Chambazone.com in category: Blog sharing the story of making money online. You will find the answer right below.
Keep Reading
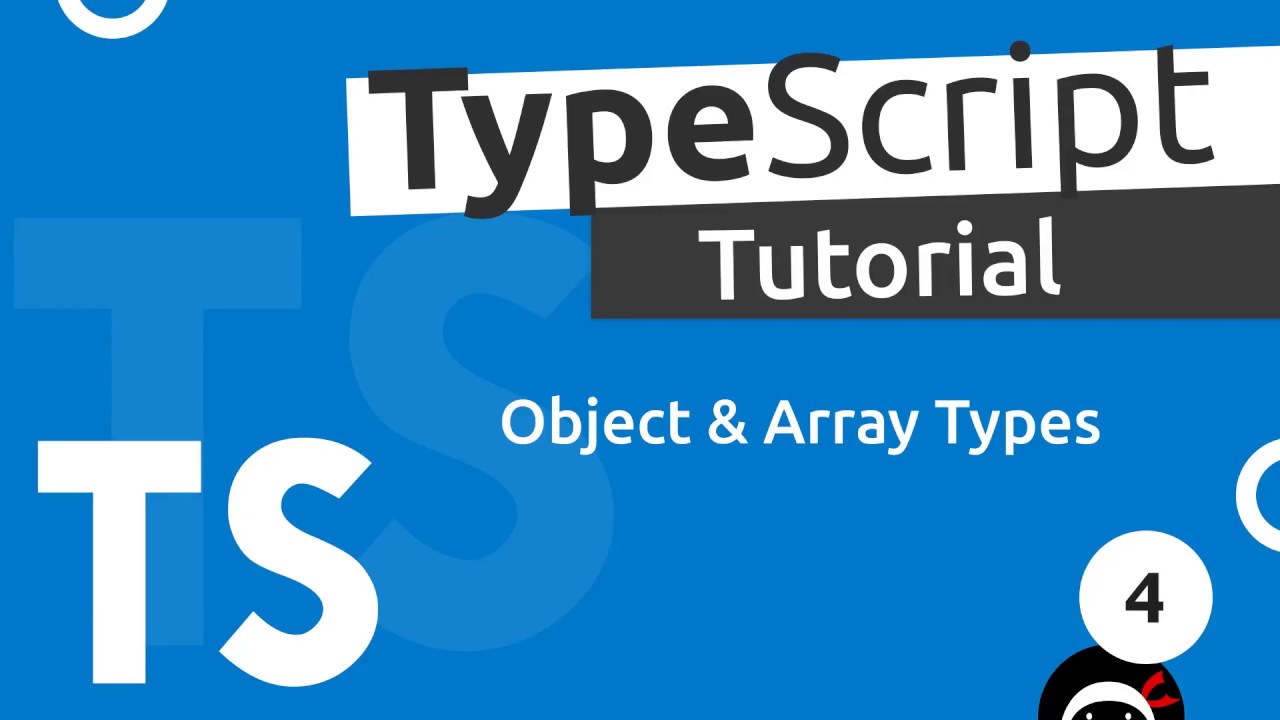
How do I use key values in TypeScript?
Use an index signature to define a key-value pair in TypeScript, e.g. const employee: { [key: string]: string | number } = {} . An index signature is used when we don’t know all the names of a type’s keys ahead of time, but we know the shape of their values. Copied!
What is key value pair in TypeScript?
A key-value pair is a wonderful functionality in an object-oriented programming approach that can be used in TypeScript for generating values. These key-value pairs in TypeScript are present in TypeScript Object. The values can be function, array of objects, etc.
TypeScript Tutorial #4 – Objects Arrays
Images related to the topicTypeScript Tutorial #4 – Objects Arrays
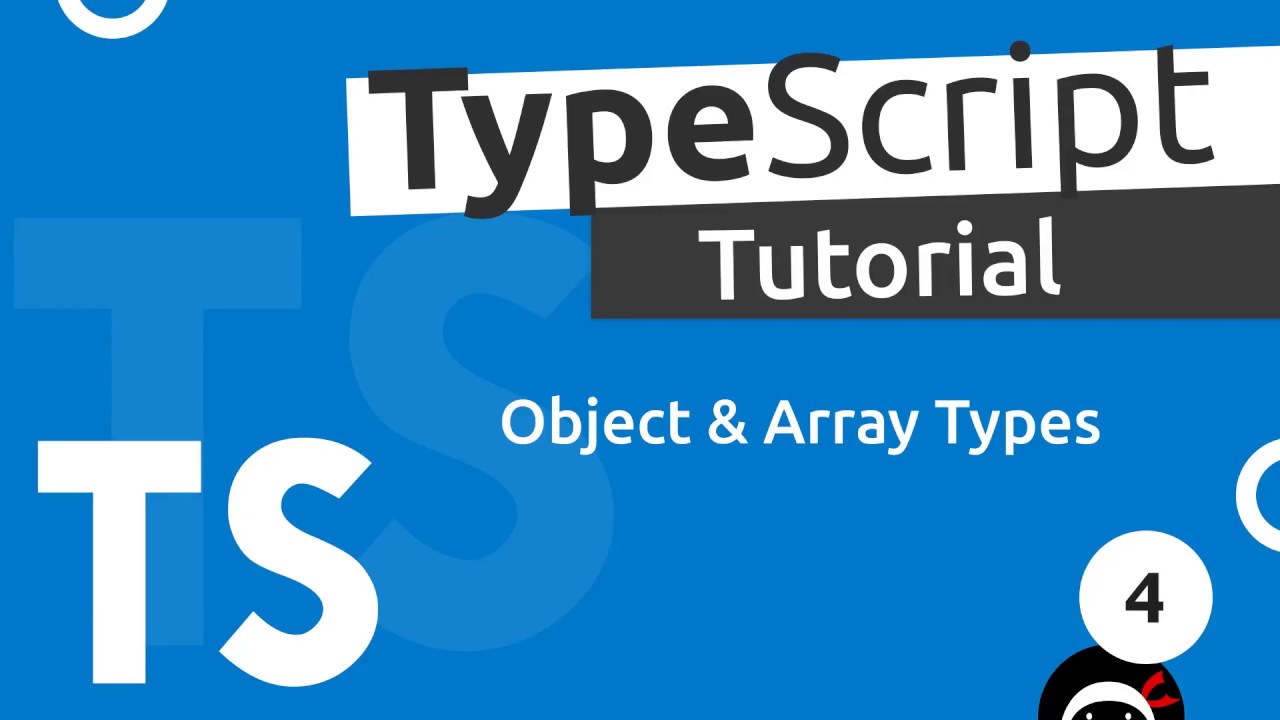
How do I add a key value pair in TypeScript?
- let indexedArray: {[key: string]: number}
-
- let indexedArray: {[key: string]: number} = {
- foo: 123,
- bar: 456.
- }
-
- indexedArray[‘foo’] = 12;
How do you find the value of an object in TypeScript?
- Use the Object. keys() method to get an array of the object’s keys.
- Type the array to be an array of the object’s keys.
- Use the find() method to get the key by its value.
What is key string in TypeScript?
The {[key: string]: string} syntax is an index signature in TypeScript and is used when we don’t know all the names of a type’s properties ahead of time, but know the shape of the values. The index signature in the examples means that when an the object is indexed with a string , it will return a string .
What is key in TypeScript?
This is a key/value structure, named index signatures (or previously known as indexable Types) in typescript. The key is a string and the value is a boolean . For example: let map : { [key: string]: boolean} = {}; map[“foo”] = true; map[“bar”] = false; map.
What is key-value pair in Javascript?
An object contains properties, or key-value pairs. The desk object above has four properties. Each property has a name, which is also called a key, and a corresponding value. For instance, the key height has the value “4 feet” . Together, the key and value make up a single property.
See some more details on the topic typescript key value list here:
Typescript Key Value Pair | Internal Working and Advantages
A key-value pair is a wonderful functionality in an object-oriented programming approach that can be used in TypeScript for generating values. These key-value …
Defining and using Key-Value pair in TypeScript | bobbyhadz
Use an index signature to define a key-value pair in TypeScript, e.g. const employee: { [key: string]: string | number } = {} .
typescript key value array Code Example – Grepper
“typescript key value array” Code Answer’s ; 1. const keyOptions = [“name”, “gender”, “address”] as const; ; 2. type TCustomKeys = { [key in …
Is key-value pair available in Typescript? – SyntaxFix
Is key-value pair available in Typescript? Yes. Called an index signature: interface Foo { [key: string]: Bar; } let foo:Foo = {};.
What is an index signature TypeScript?
In typescript, Index Signature identifies key type for indexing of an object. Everytime an object in typescript is created and indexing is expected on that object then developers must specify Index Signature .
How do I use maps in TypeScript?
…
Map methods.
SN | Methods | Descriptions |
---|---|---|
1. | map.set(key, value) | It is used to add entries in the map. |
How do you push values into objects in TypeScript?
…
Push an object to an Array in TypeScript #
- Set the type of the array to Type[] .
- Set the type of the object to Type .
- Use the push() method to push the object to the array.
How do I add a new property to an existing object in TypeScript?
To add a property to an object in TypeScript, set the property as optional on the interface you assign to the object using a question mark. You can then add the property at a later point in time without getting a type error. Copied!
How do you push the value of a object?
- var element = {}, cart = [];
- element. id = id;
- element. quantity = quantity;
- cart. push(element);
- var element = {}, cart = [];
- element. id = id;
- element. quantity = quantity;
- cart. push({element: element});
JavaScript ES6 Features: Creating Key/Value Pairs with Maps
Images related to the topicJavaScript ES6 Features: Creating Key/Value Pairs with Maps
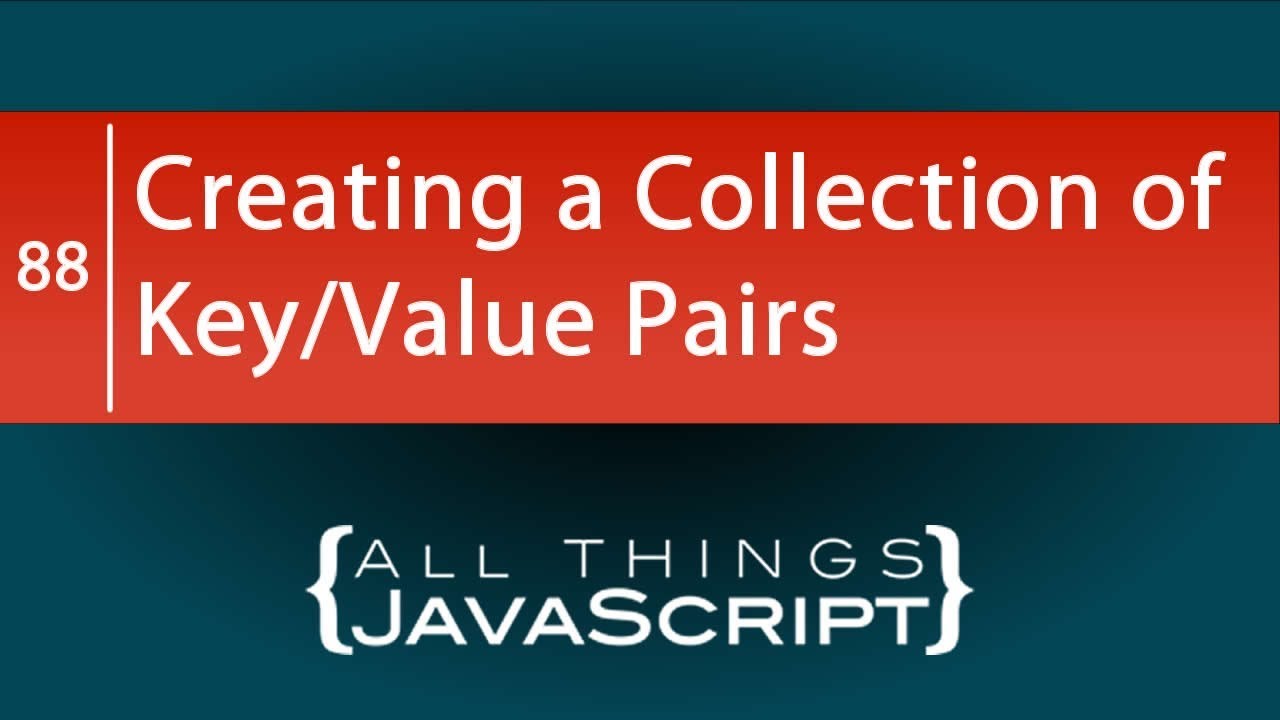
How do you find the value of an array of objects?
- Using Array. prototype. find() function. …
- Using Array. prototype. findIndex() function. …
- Using Array. prototype. forEach() function. …
- Using Array. prototype. …
- Using jQuery. The jQuery’s $. …
- Using Lodash/Underscore Library. The Underscore and Lodash library have the _.
How do you access an array of objects?
A nested data structure is an array or object which refers to other arrays or objects, i.e. its values are arrays or objects. Such structures can be accessed by consecutively applying dot or bracket notation. Here is an example: const data = { code: 42, items: [{ id: 1, name: ‘foo’ }, { id: 2, name: ‘bar’ }] };
How do I iterate over an object in JavaScript?
- const items = { ‘first’: new Date(), ‘second’: 2, ‘third’: ‘test’ }
- items. map(item => {})
- items. forEach(item => {})
- for (const item of items) {}
- for (const item in items) { console. log(item) }
- Object. entries(items). map(item => { console. log(item) }) Object.
How do you change the key name of an object in TypeScript?
- obj = { name: ‘Bobo’ } //key: value.
- obj. newName = obj. name // on object create new key name. Assign old value to this.
- delete obj. name //delete object with old key name.
Can we use string as key in HashMap?
String is as a key of the HashMap
When you pass the key to retrieve its value, the hash code is calculated again, and the value in the position represented by the hash code is fetched (if both hash codes are equal).
What are mapped types in TypeScript?
Mapped types are a handy TypeScript feature that allow authors to keep their types DRY (“Don’t Repeat Yourself”). However, because they toe the line between programming and metaprogramming, they can be difficult to understand at first.
How do you get the key of a type?
To get the keys of a TypeScript interface as array of strings, we can use the keyof keyword. interface Person { name: string; age: number; location: string; } type Keys = keyof Person; to create the Keys type by setting it to keyof Person .
What is a key string?
KeyString ( _KeyCode_ , _KeyCode2_ ) expression A variable that represents an Application object.
How do you define an array of objects in TypeScript?
One of which is Array of Objects, in TypeScript, the user can define an array of objects by placing brackets after the interface. It can be named interface or an inline interface. Let us, deep-dive, into the Syntax description for declaring Array of Objects in TypeScript and explore a few examples.
How can I get key and value from JSON object in typescript?
- myObject = {
- “key”: “value”
- }
-
- Object. keys(myObject); // get array of keys.
Mapped Types – Advanced TypeScript
Images related to the topicMapped Types – Advanced TypeScript
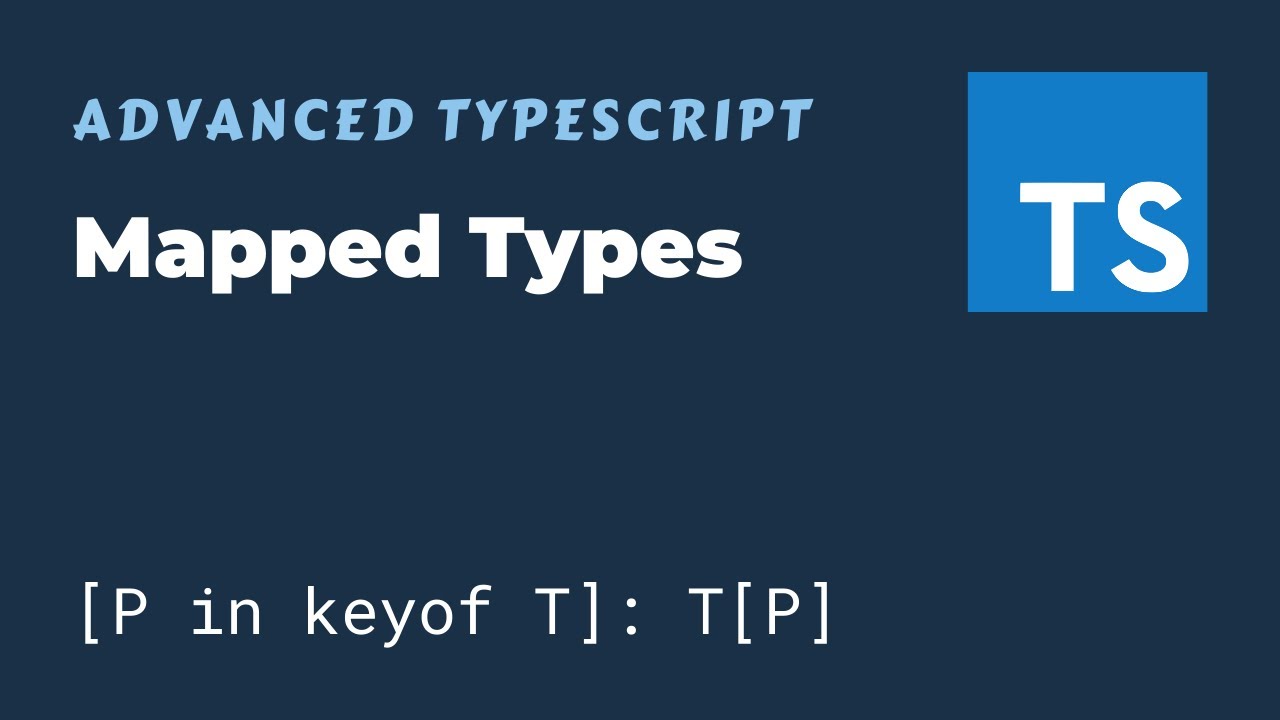
How do you add a key-value pair in JavaScript?
- Use bracket [] notation, e.g. obj[‘name’] = ‘John’ .
- Use dot . notation, e.g. obj.name = ‘John’ .
- Use the Object. assign() method, passing it a target and source objects as parameters.
How can store data in key-value pair in JavaScript?
- var myArray = {id1: 100, id2: 200, “tag with spaces”: 300};
- myArray. id3 = 400;
- myArray[“id4”] = 500;
-
- for (var key in myArray) {
- console. log(“key ” + key + ” has value ” + myArray[key]);
Related searches to typescript key value list
- typescript object to key value array
- typescript key-value dictionary
- typescript get list of keys
- typescript key value map
- typescript value of key
- typescript key-value type
- convert list to key value pair typescript
- typescript list of keys
- typescript key value dictionary
- typescript key value interface
- typescript key value pair foreach
- typescript key-value
- typescript convert key value pair to object
- typescript key value type
Information related to the topic typescript key value list
Here are the search results of the thread typescript key value list from Bing. You can read more if you want.
You have just come across an article on the topic typescript key value list. If you found this article useful, please share it. Thank you very much.